SSM框架分页展示Hdfs文件列表,返回上一级,进入下一级,上传文件,删除文件,下载文件前后端代码
时间: 2023-08-31 20:26:53 浏览: 74
### 回答1:
由于HDFS是一个分布式文件系统,它不支持直接的文件分页展示。因此,我们需要使用Hadoop的API来获取文件列表,并在后端进行分页处理。以下是一个简单的SSM框架分页展示HDFS文件列表的示例代码,包括返回上一级,进入下一级,上传文件,删除文件,下载文件的前后端代码。
前端代码:
```
<!-- 文件列表展示 -->
<table id="fileListTable" class="table table-bordered table-hover">
<thead>
<tr>
<th>文件名</th>
<th>大小</th>
<th>修改时间</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<!-- 文件列表数据由后台填充 -->
</tbody>
</table>
<!-- 分页控件 -->
<div class="text-center">
<ul class="pagination">
<li><a href="#" onclick="toPrevPage()">«</a></li>
<li><a href="#" onclick="toNextPage()">»</a></li>
</ul>
</div>
<!-- 上传文件模态框 -->
<div id="uploadFileModal" class="modal fade" tabindex="-1" role="dialog" aria-labelledby="uploadFileModalLabel" aria-hidden="true">
<div class="modal-dialog">
<div class="modal-content">
<form id="uploadFileForm" method="post" enctype="multipart/form-data">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal" aria-hidden="true">×</button>
<h4 class="modal-title" id="uploadFileModalLabel">上传文件</h4>
</div>
<div class="modal-body">
<div class="form-group">
<label for="file">选择文件:</label>
<input type="file" name="file" id="file">
</div>
<div class="form-group">
<label for="path">上传路径:</label>
<input type="text" name="path" id="path" class="form-control">
</div>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-default" data-dismiss="modal">取消</button>
<button type="submit" class="btn btn-primary">上传</button>
</div>
</form>
</div>
</div>
</div>
<!-- 删除文件模态框 -->
<div id="deleteFileModal" class="modal fade" tabindex="-1" role="dialog" aria-labelledby="deleteFileModalLabel" aria-hidden="true">
<div class="modal-dialog">
<div class="modal-content">
<form id="deleteFileForm" method="post">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal" aria-hidden="true">×</button>
<h4 class="modal-title" id="deleteFileModalLabel">删除文件</h4>
</div>
<div class="modal-body">
<div class="form-group">
<label for="deletePath">确认删除文件:</label>
<input type="text" name="path" id="deletePath" class="form-control" readonly>
</div>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-default" data-dismiss="modal">取消</button>
<button type="submit" class="btn btn-danger">删除</button>
</div>
</form>
</div>
</div>
</div>
<script type="text/javascript">
// 当前页码
var currentPage = 1;
// 分页大小
var pageSize = 10;
// 文件列表总页数
var totalPage = 1;
// 获取文件列表
function getFileList(path) {
$.get("getFileList", {"path": path, "currentPage": currentPage, "pageSize": pageSize}, function (result) {
var fileList = result.fileList;
var html = "";
for (var i = 0; i < fileList.length; i++) {
var file = fileList[i];
html += "<tr>";
html += "<td>" + file.name + "</td>";
html += "<td>" + file.size + "</td>";
html += "<td>" + file.modifyTime + "</td>";
html += "<td>";
html += "<button class='btn btn-default' onclick='downloadFile(\"" + file.path + "\")'>下载</button>";
html += "<button class='btn btn-danger' onclick='showDeleteFileModal(\"" + file.path + "\")'>删除</button>";
html += "</td>";
html += "</tr>";
}
$("#fileListTable tbody").html(html);
totalPage = result.totalPage;
$("#fileListTable_paginate span").html("第" + currentPage + "页,共" + totalPage + "页");
});
}
// 返回上一级
function toPrevPage() {
if (currentPage > 1) {
currentPage--;
getFileList($("#path").val());
}
}
// 进入下一级
function toNextPage() {
if (currentPage < totalPage) {
currentPage++;
getFileList($("#path").val());
}
}
// 显示上传文件模态框
function showUploadFileModal() {
$("#uploadFileForm")[0].reset();
$("#uploadFileModal").modal("show");
}
// 上传文件
function uploadFile() {
var formData = new FormData($("#uploadFileForm")[0]);
$.ajax({
url: "uploadFile",
type: "POST",
data: formData,
dataType: "json",
contentType: false,
processData: false,
success: function (result) {
if (result.code == 0) {
alert("上传成功!");
$("#uploadFileModal").modal("hide");
getFileList($("#path").val());
} else {
alert("上传失败:" + result.msg);
}
},
error: function () {
alert("上传失败!");
}
});
}
// 显示删除文件模态框
function showDeleteFileModal(path) {
$("#deletePath").val(path);
$("#deleteFileModal").modal("show");
}
// 删除文件
function deleteFile() {
$.post("deleteFile", {"path": $("#deletePath").val()}, function (result) {
if (result.code == 0) {
alert("删除成功!");
$("#deleteFileModal").modal("hide");
getFileList($("#path").val());
} else {
alert("删除失败:" + result.msg);
}
});
}
// 下载文件
function downloadFile(path) {
window.location.href = "downloadFile?path=" + encodeURIComponent(path);
}
// 页面加载完成后获取文件列表
$(function () {
getFileList("/");
});
</script>
```
后端代码:
```
/**
* 获取文件列表
* @param path 文件路径
* @param currentPage 当前页码
* @param pageSize 分页大小
* @return 文件列表和分页信息
*/
@RequestMapping("/getFileList")
@ResponseBody
public Object getFileList(String path, int currentPage, int pageSize) {
try {
// 获取文件系统
Configuration conf = new Configuration();
conf.set("fs.defaultFS", "hdfs://localhost:9000");
FileSystem fs = FileSystem.get(conf);
// 获取文件列表
FileStatus[] fileStatuses = fs.listStatus(new Path(path));
// 封装文件列表数据
List<Map<String, Object>> fileList = new ArrayList<>();
for (FileStatus fileStatus : fileStatuses) {
Map<String, Object> file = new HashMap<>();
file.put("name", fileStatus.getPath().getName());
file.put("size", FileUtils.byteCountToDisplaySize(fileStatus.getLen()));
file.put("modifyTime", new SimpleDateFormat("yyyy-MM-dd HH:mm:ss").format(new Date(fileStatus.getModificationTime())));
file.put("path", fileStatus.getPath().toString());
fileList.add(file);
}
// 分页处理
int totalPage = (int) Math.ceil((double) fileList.size() / pageSize);
int endIndex = currentPage * pageSize;
if (endIndex > fileList.size()) {
endIndex = fileList.size();
}
List<Map<String, Object>> pagedFileList = fileList.subList((currentPage - 1) * pageSize, endIndex);
// 封装分页信息
Map<String, Object> result = new HashMap<>();
result.put("fileList", pagedFileList);
result.put("totalPage", totalPage);
return result;
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
/**
* 上传文件
* @param file 上传的文件
* @param path 上传路径
* @return
*/
@RequestMapping("/uploadFile")
@ResponseBody
public Object uploadFile(@RequestParam("file") MultipartFile file, String path) {
try {
// 获取文件系统
Configuration conf = new Configuration();
conf.set("fs.defaultFS", "hdfs://localhost:9000");
FileSystem fs = FileSystem.get(conf);
// 上传文件
String fileName = file.getOriginalFilename();
String destPath = path + "/" + fileName;
Path dest = new Path(destPath);
FSDataOutputStream outputStream = fs.create(dest);
InputStream inputStream = file.getInputStream();
IOUtils.copy(inputStream, outputStream);
IOUtils.closeQuietly(inputStream);
IOUtils.closeQuietly(outputStream);
// 返回上传成功信息
Map<String, Object> result = new HashMap<>();
result.put("code", 0);
return result;
} catch (Exception e) {
e.printStackTrace();
// 返回上传失败信息
Map<String, Object> result = new HashMap<>();
result.put("code", -1);
result.put("msg", e.getMessage());
return result;
}
}
/**
* 删除文件
* @param path 文件路径
* @return
*/
@RequestMapping("/deleteFile")
@ResponseBody
public Object deleteFile(String path) {
try {
// 获取文件系统
Configuration conf = new Configuration();
conf.set("fs.defaultFS", "hdfs://localhost:9000");
FileSystem fs = FileSystem.get(conf);
// 删除文件
Path deletePath = new Path(path);
fs.delete(deletePath, true);
// 返回删除成功信息
Map<String, Object> result = new HashMap<>();
result.put("code", 0);
return result;
} catch (Exception e) {
e.printStackTrace();
// 返回删除失败信息
Map<String, Object> result = new HashMap<>();
result.put("code", -1);
result.put("msg", e.getMessage());
return result;
}
}
/**
* 下载文件
* @param response
* @param path 文件路径
*/
@RequestMapping("/downloadFile")
public void downloadFile(HttpServletResponse response, String path) {
try {
// 获取文件系统
Configuration conf = new Configuration();
conf.set("fs.defaultFS", "hdfs://localhost:9000");
FileSystem fs = FileSystem.get(conf);
// 下载文件
Path downloadPath = new Path(path);
FSDataInputStream inputStream = fs.open(downloadPath);
OutputStream outputStream = response.getOutputStream();
response.setContentType("application/octet-stream");
response.setHeader("Content-Disposition", "attachment;filename=" + URLEncoder.encode(downloadPath.getName(), "UTF-8"));
IOUtils.copy(inputStream, outputStream);
IOUtils.closeQuietly(inputStream);
IOUtils.closeQuietly(outputStream);
} catch (Exception e) {
e.printStackTrace();
}
}
```
### 回答2:
要实现SSM框架下对HDFS文件列表的分页展示以及相关操作,可以考虑以下步骤:
1. 前端代码:
在前端页面中,可以通过一个文件列表展示的表格来展示HDFS文件列表。可以使用HTML和CSS来创建表格结构,并通过JavaScript实现动态渲染数据。
同时,需要添加上一页和下一页的按钮,以及上传文件、删除文件和下载文件的按钮。
2. 后端代码:
在后端中,需要使用Java编写相应的Controller接口和Service类。
- 分页展示文件列表:
在Controller中,可以使用@RequestMapping注解来定义相应的URL地址,并在其中调用Service类中的方法来获取分页数据。
Service类中可以使用Hadoop HDFS相关API,如FileSystem类,来连接HDFS并获取文件列表信息。
最后,将获取到的数据封装成Page对象,通过ResponseEntity将数据返回给前端。
- 返回上一级和进入下一级:
在Controller中,可以通过定义相应的URL地址,并在其中调用Service类中的方法来实现返回上一级和进入下一级的操作。
Service类中可以使用Hadoop HDFS相关API,如FileSystem类的getParent和listStatus方法来实现这两个操作。
- 上传文件:
在Controller中,可以使用@RequestMapping注解来定义相应的URL地址,并在其中调用Service类中的方法来实现上传文件的操作。
Service类中可以使用Hadoop HDFS相关API,如FileSystem类的create方法来实现上传文件。
- 删除文件:
在Controller中,可以使用@RequestMapping注解来定义相应的URL地址,并在其中调用Service类中的方法来实现删除文件的操作。
Service类中可以使用Hadoop HDFS相关API,如FileSystem类的delete方法来实现删除文件。
- 下载文件:
在Controller中,可以使用@RequestMapping注解来定义相应的URL地址,并在其中调用Service类中的方法来实现下载文件的操作。
Service类中可以使用Hadoop HDFS相关API,如FileSystem类的open方法来实现下载文件。
需要注意的是,要保证SSM框架的正常运行,需要配置好Spring、Spring MVC和MyBatis的相关配置文件,并在项目中引入相应的依赖库。
以上是实现SSM框架下对HDFS文件列表的分页展示以及相关操作的简要代码示例。具体的实现还需要根据项目需求和具体情况进行进一步的开发和调试。
### 回答3:
SSM框架是指Spring+SpringMVC+MyBatis的组合,用于快速开发Java Web应用程序。要实现在SSM框架中分页展示Hadoop分布式文件系统(HDFS)文件列表,并提供返回上一级、进入下一级、上传文件、删除文件和下载文件的功能,需要编写前后端代码。
1. 后端代码:
首先,在Spring MVC中配置相关的Controller和RequestMapping,用于处理前端请求。其中:
- 使用MyBatis框架访问HDFS文件系统,通过Java API获取HDFS文件列表、上一级目录、下一级目录。
- 通过HDFS文件系统API实现文件的上传、删除和下载功能。
2. 前端代码:
使用HTML、CSS和JavaScript等前端技术,通过AJAX调用后端接口实现与后端的交互,实现以下功能:
- 分页展示HDFS文件列表,通过发送带有分页参数的请求获取指定页的文件列表,并将结果渲染到页面。
- 点击文件夹进入下一级目录,发送带有目录路径参数的请求获取该目录下的文件列表,并将结果渲染到页面。
- 点击返回上一级按钮,发送请求获取上一级目录的文件列表,并将结果渲染到页面。
- 点击上传文件按钮,选择本地文件后将其通过AJAX请求发送给后端进行文件上传。
- 点击删除文件按钮,发送带有文件路径参数的请求给后端进行文件删除操作。
- 点击下载文件按钮,发送带有文件路径参数的请求给后端,后端将文件内容返回给前端,前端通过JavaScript实现文件下载。
通过以上前后端代码的编写和协作,就能实现在SSM框架下分页展示HDFS文件列表,以及提供返回上一级、进入下一级、上传文件、删除文件和下载文件的功能。
相关推荐
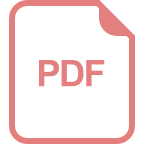
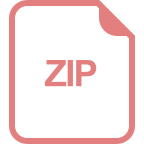
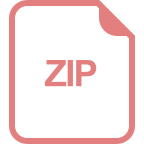
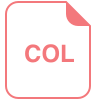
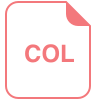
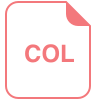
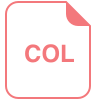
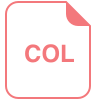



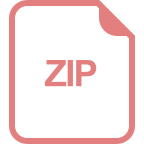
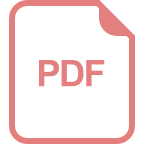
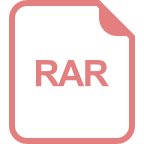
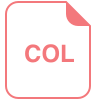
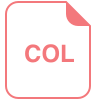
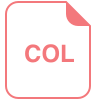
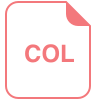