分别用字符数组和字符指针作为函数参数,编写下列字符串处理函数,并给出测试结果: (1)求字符串长度的函数strLen(源字符串); (2)字符串复制函数strCpy(源字符串,目的字符串); (3)字符串连接函数strCat(字符串1,字符串2):字符串2连接到字符串1中; (4)字符串比较大小函数strCmp(字符串1,字符串2):若字符串1>字符串2,返回值大于0,比如1,若字符串1<字符串2,返回值小于0,如-1,若字符串1=字符串2,返回值等于0。
时间: 2023-11-27 17:13:46 浏览: 97
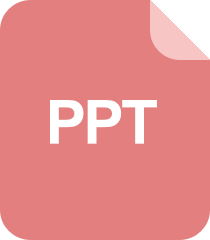
字符串处理函数

好的,我会根据您的要求回答您的问题。
(1)使用字符数组作为函数参数,求字符串长度的函数strLen:
```c++
int strLen(char s[]) {
int len = 0;
for (int i = 0; s[i] != '\0'; i++) {
len++;
}
return len;
}
```
测试代码:
```c++
char s[] = "hello world";
printf("字符串长度为:%d\n", strLen(s));
```
输出结果:字符串长度为:11
(2)使用字符指针作为函数参数,字符串复制函数strCpy:
```c++
void strCpy(char* dest, const char* src) {
while (*src != '\0') {
*dest = *src;
dest++;
src++;
}
*dest = '\0';
}
```
测试代码:
```c++
char s1[] = "hello";
char s2[10];
strCpy(s2, s1);
printf("复制后的字符串为:%s\n", s2);
```
输出结果:复制后的字符串为:hello
(3)使用字符数组作为函数参数,字符串连接函数strCat:
```c++
void strCat(char s1[], const char s2[]) {
int i, j;
i = j = 0;
while (s1[i] != '\0') {
i++;
}
while (s2[j] != '\0') {
s1[i] = s2[j];
i++;
j++;
}
s1[i] = '\0';
}
```
测试代码:
```c++
char s1[20] = "hello";
char s2[] = "world";
strCat(s1, s2);
printf("连接后的字符串为:%s\n", s1);
```
输出结果:连接后的字符串为:helloworld
(4)使用字符指针作为函数参数,字符串比较大小函数strCmp:
```c++
int strCmp(const char* s1, const char* s2) {
while (*s1 != '\0' && *s2 != '\0') {
if (*s1 > *s2) {
return 1;
} else if (*s1 < *s2) {
return -1;
}
s1++;
s2++;
}
if (*s1 == '\0' && *s2 == '\0') {
return 0;
} else if (*s1 == '\0') {
return -1;
} else {
return 1;
}
}
```
测试代码:
```c++
char s1[] = "hello";
char s2[] = "hello world";
printf("比较结果为:%d\n", strCmp(s1, s2));
```
输出结果:比较结果为:-1
阅读全文
相关推荐
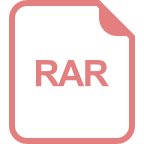
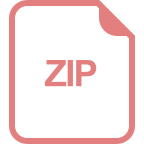
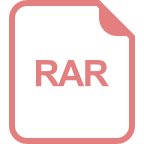
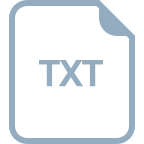
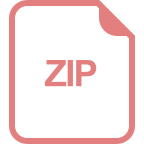
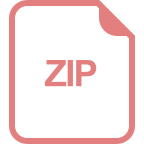
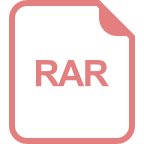
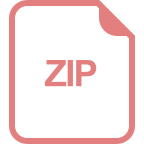
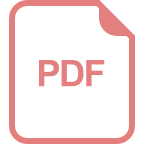
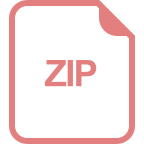
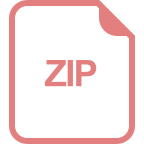
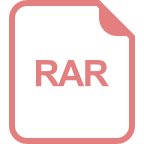
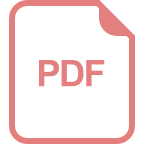
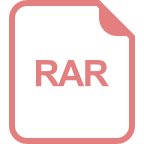
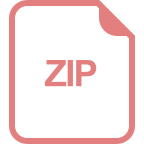
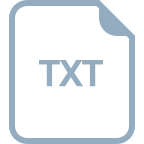