什么是implicit representation类神经网络?DNN属于CNN网络的一种吗?如何用python写DNN网络和CNN以及MLP网络
时间: 2023-05-13 16:04:44 浏览: 174
Implicit representation neural networks are a type of neural network that can learn to represent complex, high-dimensional data without the need for explicit feature engineering. DNNs (Deep Neural Networks) are a type of neural network that can have multiple hidden layers, and they can be used for a variety of tasks, including image classification, speech recognition, and natural language processing. CNNs (Convolutional Neural Networks) are a type of DNN that are particularly well-suited for image processing tasks, as they can learn to recognize patterns in images. MLPs (Multilayer Perceptrons) are another type of DNN that can be used for a variety of tasks, including classification and regression.
To write a DNN in Python, you can use a deep learning library such as TensorFlow or PyTorch. Here is an example of how to create a simple DNN using TensorFlow:
```
import tensorflow as tf
# Define the model architecture
model = tf.keras.Sequential([
tf.keras.layers.Dense(64, activation='relu', input_shape=(784,)),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
# Compile the model
model.compile(optimizer=tf.keras.optimizers.Adam(0.01),
loss='categorical_crossentropy',
metrics=['accuracy'])
# Train the model
model.fit(x_train, y_train, epochs=10, batch_size=32, validation_data=(x_val, y_val))
```
To write a CNN in Python, you can use a similar approach, but with convolutional layers instead of fully connected layers. Here is an example of how to create a simple CNN using TensorFlow:
```
import tensorflow as tf
# Define the model architecture
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, (3, 3), activation='relu', input_shape=(28, 28, 1)),
tf.keras.layers.MaxPooling2D((2, 2)),
tf.keras.layers.Conv2D(64, (3, 3), activation='relu'),
tf.keras.layers.MaxPooling2D((2, 2)),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
# Compile the model
model.compile(optimizer=tf.keras.optimizers.Adam(0.01),
loss='categorical_crossentropy',
metrics=['accuracy'])
# Train the model
model.fit(x_train, y_train, epochs=10, batch_size=32, validation_data=(x_val, y_val))
```
To write an MLP in Python, you can use a similar approach to the DNN example above, but with only fully connected layers. Here is an example of how to create a simple MLP using TensorFlow:
```
import tensorflow as tf
# Define the model architecture
model = tf.keras.Sequential([
tf.keras.layers.Dense(64, activation='relu', input_shape=(784,)),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
# Compile the model
model.compile(optimizer=tf.keras.optimizers.Adam(0.01),
loss='categorical_crossentropy',
metrics=['accuracy'])
# Train the model
model.fit(x_train, y_train, epochs=10, batch_size=32, validation_data=(x_val, y_val))
```
阅读全文
相关推荐








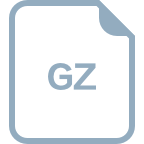

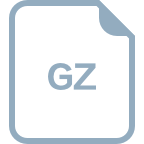







