pytorch编写文本二分类RNN代码
时间: 2023-07-07 12:38:33 浏览: 119
以下是一个使用 PyTorch 编写的文本二分类 RNN 代码示例:
```python
import torch
import torch.nn as nn
class TextRNN(nn.Module):
def __init__(self, vocab_size, embedding_dim, hidden_dim, output_dim, n_layers, bidirectional, dropout):
super().__init__()
self.embedding = nn.Embedding(vocab_size, embedding_dim)
self.rnn = nn.LSTM(embedding_dim, hidden_dim, num_layers=n_layers, bidirectional=bidirectional, dropout=dropout)
self.fc = nn.Linear(hidden_dim * 2 if bidirectional else hidden_dim, output_dim)
self.dropout = nn.Dropout(dropout)
def forward(self, text, text_lengths):
embedded = self.dropout(self.embedding(text))
packed_embedded = nn.utils.rnn.pack_padded_sequence(embedded, text_lengths.to('cpu'), enforce_sorted=False)
packed_output, (hidden, cell) = self.rnn(packed_embedded)
output, output_lengths = nn.utils.rnn.pad_packed_sequence(packed_output)
hidden = self.dropout(torch.cat((hidden[-2, :, :], hidden[-1, :, :]), dim=1)) if self.rnn.bidirectional else self.dropout(hidden[-1, :, :])
return self.fc(hidden)
```
该模型使用了 LSTM 层,并使用了 PyTorch 提供的 `pack_padded_sequence` 和 `pad_packed_sequence` 函数,来处理变长的文本序列。模型的输入为一个文本序列和该序列的长度,输出为两个类别的概率。
阅读全文
相关推荐
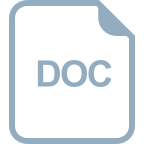
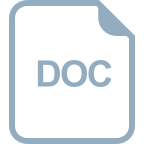
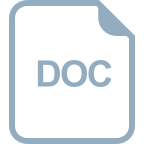
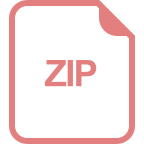
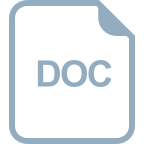
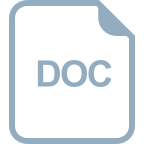
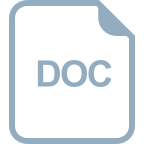
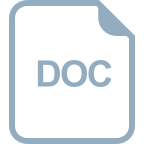
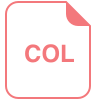
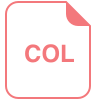
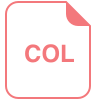
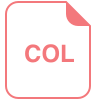
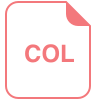
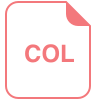
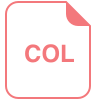
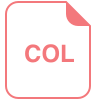
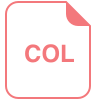
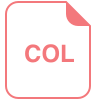
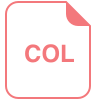