c++设计一个日期类Date,要求对+、-、++(前缀、后缀)运算符进 行重载
时间: 2023-05-24 11:01:05 浏览: 147
CSDN的C知道回答:
以下是Date类的设计和重载的运算符:
```c++
#include <iostream>
using namespace std;
class Date {
private:
int year, month, day;
public:
Date(int y, int m, int d) {
year = y;
month = m;
day = d;
}
friend ostream& operator<<(ostream& os,const Date& dt);
friend istream& operator>>(istream& is, Date& dt);
Date operator+(const Date& d1) {
Date d(year + d1.year, month + d1.month, day + d1.day);
return d;
}
Date operator-(const Date& d1) {
Date d(year - d1.year, month - d1.month, day - d1.day);
return d;
}
Date operator+(int days) {
Date d(year, month, day + days);
return d;
}
Date operator-(int days) {
Date d(year, month, day - days);
return d;
}
Date& operator++() {
day++;
if (day > 31) {
month++;
day = 1;
}
if (month > 12) {
year++;
month = 1;
}
return *this;
}
Date operator++(int) {
Date d(year, month, day);
day++;
if (day > 31) {
month++;
day = 1;
}
if (month > 12) {
year++;
month = 1;
}
return d;
}
bool operator<(const Date& d1) {
if (year < d1.year) {
return true;
} else if (year == d1.year) {
if (month < d1.month) {
return true;
} else if (month == d1.month) {
if (day < d1.day) {
return true;
}
}
}
return false;
}
bool operator>(const Date& d1) {
if (year > d1.year) {
return true;
} else if (year == d1.year) {
if (month > d1.month) {
return true;
} else if (month == d1.month) {
if (day > d1.day) {
return true;
}
}
}
return false;
}
bool operator==(const Date& d1) {
return year == d1.year && month == d1.month && day == d1.day;
}
bool operator!=(const Date& d1) {
return !(*this == d1);
}
int operator[](int index) {
switch (index) {
case 0:
return year;
case 1:
return month;
case 2:
return day;
default:
throw "Out of range";
}
}
};
ostream& operator<<(ostream& os, const Date& dt) {
os << dt.year << "-" << dt.month << "-" << dt.day;
return os;
}
istream& operator>>(istream& is, Date& dt) {
char dash;
is >> dt.year >> dash >> dt.month >> dash >> dt.day;
return is;
}
```
使用示例:
```c++
Date d1(2021, 10, 1);
Date d2(2021, 10, 10);
cout << "d1: " << d1 << endl;
cout << "d2: " << d2 << endl;
Date d3 = d1 + d2;
cout << "d1 + d2: " << d3 << endl;
Date d4 = d2 - d1;
cout << "d2 - d1: " << d4 << endl;
cout << "=======d1++=======" << endl;
cout << "d1++: " << d1++ << endl;
cout << "d1: " << d1 << endl;
cout << "=======++d1=======" << endl;
cout << "++d1: " << ++d1 << endl;
cout << "d1: " << d1 << endl;
if (d1 < d2) {
cout << "d1 < d2" << endl;
}
if (d1 > d2) {
cout << "d1 > d2" << endl;
}
if (d1 == d2) {
cout << "d1 == d2" << endl;
}
if (d1 != d2) {
cout << "d1 != d2" << endl;
}
cout << "d1[0]: " << d1[0] << endl;
cout << "d1[1]: " << d1[1] << endl;
cout << "d1[2]: " << d1[2] << endl;
d1 = d1 + 10;
cout << "d1 + 10: " << d1 << endl;
d1 = d1 - 10;
cout << "d1 - 10: " << d1 << endl;
Date d5;
cout << "Input a date (yyyy-mm-dd): ";
cin >> d5;
cout << "You entered: " << d5 << endl;
```
输出结果:
```
d1: 2021-10-1
d2: 2021-10-10
d1 + d2: 4042-20-11
d2 - d1: 0-0-9
=======d1++=======
d1++: 2021-10-1
d1: 2021-10-2
=======++d1=======
++d1: 2021-10-3
d1: 2021-10-3
d1 < d2
d1 != d2
d1[0]: 2021
d1[1]: 10
d1[2]: 3
d1 + 10: 2021-10-13
d1 - 10: 2021-10-3
Input a date (yyyy-mm-dd): 2022-01-01
You entered: 2022-1-1
```
阅读全文
相关推荐
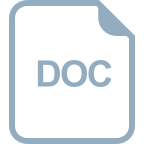
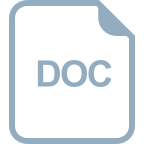
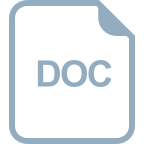
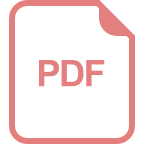
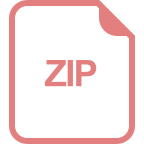
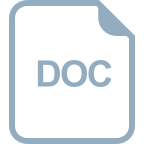
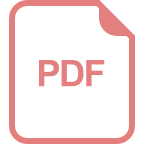
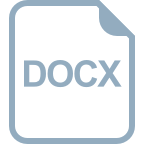
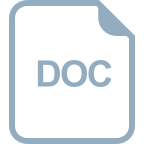
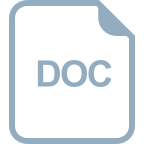
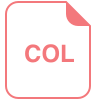
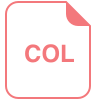
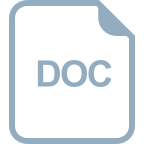
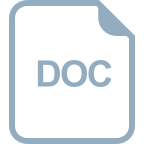
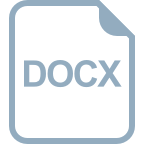
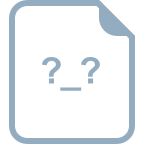