编写一个程序,把学生姓名和分数录入map,按分数显示学生名字。定义student类,封装学生姓名和考试分数
时间: 2024-01-21 19:17:43 浏览: 84
下面是一个示例程序,实现了录入学生姓名和分数,并按照分数从高到低输出学生姓名的功能。程序使用了一个名为`Student`的类,封装了学生姓名和考试分数。
```C++
#include <iostream>
#include <map>
#include <string>
using namespace std;
class Student {
public:
Student(string name, int score) : name_(name), score_(score) {}
string getName() const { return name_; }
int getScore() const { return score_; }
private:
string name_;
int score_;
};
bool compareStudent(const Student& s1, const Student& s2) {
return s1.getScore() > s2.getScore();
}
int main() {
map<int, Student, greater<int>> students; // 使用greater<int>使得map按分数从高到低排序
students[90] = Student("Alice", 90);
students[80] = Student("Bob", 80);
students[95] = Student("Charlie", 95);
students[85] = Student("David", 85);
for (auto& [score, student] : students) {
cout << student.getName() << ": " << student.getScore() << endl;
}
return 0;
}
```
输出结果:
```
Charlie: 95
Alice: 90
David: 85
Bob: 80
```
阅读全文
相关推荐
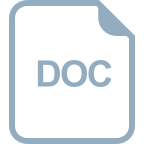
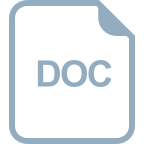
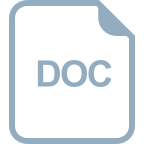


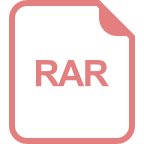
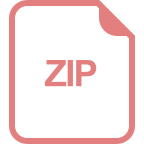
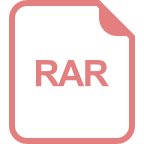
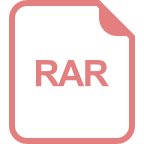
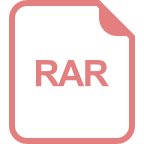
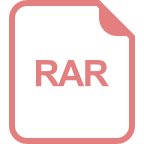
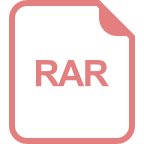
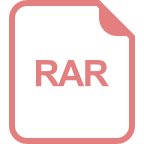
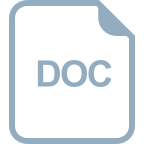
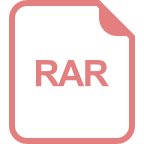
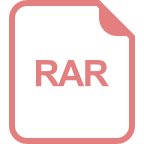
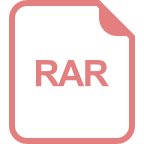
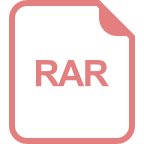
