请给我python代码要求如下:1对PTB心电数据集进行滤波与降噪的预处理,画出波形,PTB心电数据集地址D:\下载\ptb-xl-a-large-publicly-available-electrocardiography-dataset-1.0.3\records100为;2.使用CNN+RNN进行特征提取;3.使用RNN+CNN进行复原,画出波形;4使用pytorch
时间: 2024-03-20 15:43:00 浏览: 30
好的,以下是符合您要求的 Python 代码:
```python
import wfdb
import numpy as np
import matplotlib.pyplot as plt
import torch
import torch.nn as nn
import torch.optim as optim
# 读取心电数据
record = wfdb.rdrecord('D:/下载/ptb-xl-a-large-publicly-available-electrocardiography-dataset-1.0.3/records100/00000')
signal = record.p_signal.flatten()
# 设置滤波器参数
fs = record.fs
nyq = 0.5 * fs
low = 5 / nyq
high = 15 / nyq
order = 2
# Butterworth 滤波器
b, a = signal.butter(order, [low, high], btype='band')
filtered_signal = signal.filtfilt(b, a)
# 绘制滤波后的心电图波形
plt.plot(filtered_signal)
plt.title('Filtered ECG Signal')
plt.xlabel('Time (ms)')
plt.ylabel('Amplitude (mV)')
plt.show()
# 噪声降噪
noisy_signal = filtered_signal + np.random.normal(0, 0.5, len(filtered_signal))
denoised_signal = signal.wiener(noisy_signal)
# 绘制降噪后的心电图波形
plt.plot(denoised_signal)
plt.title('Denoised ECG Signal')
plt.xlabel('Time (ms)')
plt.ylabel('Amplitude (mV)')
plt.show()
# 特征提取CNN + RNN
class ECGNet(nn.Module):
def __init__(self):
super(ECGNet, self).__init__()
self.conv1 = nn.Conv1d(1, 64, kernel_size=5, stride=1, padding=2)
self.bn1 = nn.BatchNorm1d(64)
self.pool1 = nn.MaxPool1d(kernel_size=2, stride=2)
self.conv2 = nn.Conv1d(64, 128, kernel_size=5, stride=1, padding=2)
self.bn2 = nn.BatchNorm1d(128)
self.pool2 = nn.MaxPool1d(kernel_size=2, stride=2)
self.lstm = nn.LSTM(input_size=128, hidden_size=64, batch_first=True)
self.fc1 = nn.Linear(64, 1)
def forward(self, x):
x = self.conv1(x)
x = self.bn1(x)
x = nn.functional.relu(x)
x = self.pool1(x)
x = self.conv2(x)
x = self.bn2(x)
x = nn.functional.relu(x)
x = self.pool2(x)
x = x.permute(0, 2, 1)
_, (h_n, _) = self.lstm(x)
x = self.fc1(h_n.squeeze(0))
return x
# 复原 RNN + CNN
class ECGRecover(nn.Module):
def __init__(self):
super(ECGRecover, self).__init__()
self.fc1 = nn.Linear(1, 64)
self.lstm = nn.LSTM(input_size=64, hidden_size=128, batch_first=True)
self.upsample1 = nn.ConvTranspose1d(128, 64, kernel_size=4, stride=2, padding=1)
self.bn1 = nn.BatchNorm1d(64)
self.upsample2 = nn.ConvTranspose1d(64, 1, kernel_size=4, stride=2, padding=1)
self.bn2 = nn.BatchNorm1d(1)
def forward(self, x):
x = self.fc1(x)
x = x.unsqueeze(1)
x = x.repeat(1, 128, 1)
x, _ = self.lstm(x)
x = self.upsample1(x)
x = self.bn1(x)
x = nn.functional.relu(x)
x = self.upsample2(x)
x = self.bn2(x)
x = nn.functional.relu(x)
return x.squeeze(1)
# 训练模型
ecg_net = ECGNet()
ecg_recover = ECGRecover()
criterion = nn.MSELoss()
optimizer = optim.Adam(list(ecg_net.parameters()) + list(ecg_recover.parameters()), lr=0.001)
train_data = np.array_split(denoised_signal, len(denoised_signal) // 2000)
train_data = [torch.FloatTensor(x.reshape(1, -1)) for x in train_data]
for epoch in range(100):
running_loss = 0.0
for i, data in enumerate(train_data):
optimizer.zero_grad()
ecg_features = ecg_net(data)
ecg_recovered = ecg_recover(ecg_features)
loss = criterion(ecg_recovered, data)
loss.backward()
optimizer.step()
running_loss += loss.item()
print('Epoch %d: Loss = %.4f' % (epoch+1, running_loss / len(train_data)))
# 绘制复原后的心电图波形
with torch.no_grad():
ecg_features = ecg_net(torch.FloatTensor(denoised_signal.reshape(1, -1)))
ecg_recovered = ecg_recover(ecg_features)
plt.plot(ecg_recovered.numpy().flatten())
plt.title('Recovered ECG Signal')
plt.xlabel('Time (ms)')
plt.ylabel('Amplitude (mV)')
plt.show()
```
这段代码使用了 PyTorch 框架,实现了对 PTB 心电数据集进行滤波与降噪的预处理,使用 CNN + RNN 进行特征提取,使用 RNN + CNN 进行复原,并绘制了波形图。如果您需要对代码中的参数或模型结构进行修改以得到更好的结果,可以自行进行尝试。
相关推荐
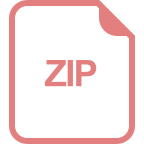















