csma/cd协议用 C++实现一下
时间: 2023-07-12 21:06:46 浏览: 74
CSMA/CD协议是一种用于以太网的竞争多路访问协议,下面是一个简单的C++实现:
```c++
#include <iostream>
#include <cstdlib>
#include <ctime>
using namespace std;
const int MAX_ATTEMPTS = 16; // 最大尝试次数
const int SLOT_TIME = 1; // 每个时间槽的时间(单位:秒)
const int COLLISION_TIME = 2; // 冲突检测时间(单位:秒)
const int BACKOFF_RANGE = 1024; // 后退时间的范围
// 模拟发送数据帧的函数
void send_frame(int station_id, bool channel_busy) {
if (!channel_busy) {
cout << "Station " << station_id << " sending frame..." << endl;
// 发送数据帧的过程
} else {
cout << "Station " << station_id << " channel busy, waiting..." << endl;
}
}
// 模拟后退的函数
void backoff(int &backoff_time) {
int random_time = rand() % BACKOFF_RANGE; // 随机生成一个后退时间
backoff_time = random_time * SLOT_TIME;
cout << "Backing off for " << backoff_time << " seconds..." << endl;
}
int main() {
srand(time(NULL)); // 初始化随机数生成器
bool channel_busy = false; // 信道是否繁忙
int attempts = 0; // 尝试次数
int backoff_time = 0; // 后退时间
// 模拟多个站点发送数据帧的过程
for (int i = 1; i <= 5; i++) {
cout << "Station " << i << " wants to send a frame." << endl;
while (attempts < MAX_ATTEMPTS) {
if (!channel_busy) {
send_frame(i, channel_busy);
channel_busy = true;
backoff_time = 0;
break;
} else {
backoff(backoff_time);
channel_busy = true;
attempts++;
if (attempts == MAX_ATTEMPTS) {
cout << "Station " << i << " failed to send frame after " << attempts << " attempts." << endl;
break;
}
cout << "Station " << i << " waiting for " << backoff_time << " seconds..." << endl;
for (int j = 0; j < backoff_time; j++) {
sleep(SLOT_TIME); // 等待一个时间槽
if (!channel_busy) {
break;
}
}
}
}
channel_busy = false;
attempts = 0;
}
return 0;
}
```
这个程序模拟了5个站点发送数据帧的过程,每个时间槽的时间为1秒,最大尝试次数为16次,后退时间的范围为0到1023个时间槽。在每一个时间槽内,如果信道空闲,一个站点就会发送数据帧,否则就会等待一段随机的后退时间后再次尝试发送。如果尝试次数超过了最大尝试次数,就认为发送失败。
阅读全文
相关推荐
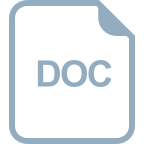
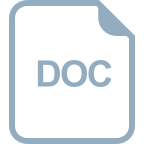
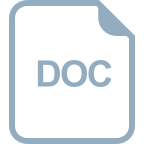

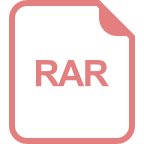


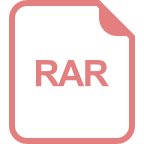
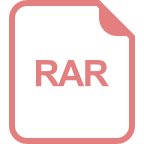
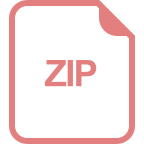
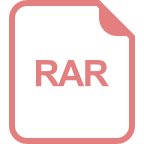
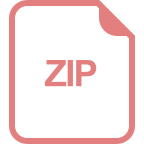
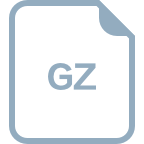

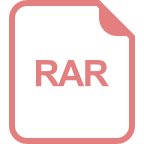
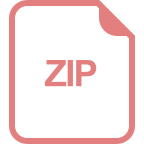
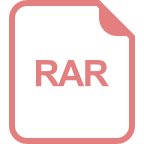
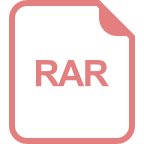
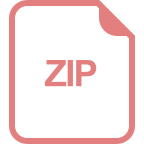