com.baomidou.mybatisplus.annotation.TableField
时间: 2023-10-08 17:08:04 浏览: 237
`@TableField` 是 MyBatis-Plus 框架中的注解,用于标注实体类中的字段与数据库表中的字段的对应关系。
例如:
```java
public class User {
@TableField("id")
private Long userId;
@TableField("name")
private String userName;
//省略getter和setter
}
```
在上述代码中,`@TableField("id")` 表示 `userId` 字段与数据库表中的 `id` 字段对应,`@TableField("name")` 表示 `userName` 字段与数据库表中的 `name` 字段对应。这样,在 MyBatis-Plus 框架中就可以使用实体类中的字段作为查询条件或者结果映射的属性。
相关问题
package com.group9.trainmanager.model; import com.baomidou.mybatisplus.annotation.IdType; import com.baomidou.mybatisplus.annotation.TableField; import com.baomidou.mybatisplus.annotation.TableId; import com.baomidou.mybatisplus.annotation.TableName; import lombok.Data; @Data @TableName("front") public class Front { @TableField("fro_id") @TableId(type = IdType.AUTO) private Integer froId; @TableField("fro_html") private String froHtml; @TableField("fro_js") private String froJs; @TableField("fro_css") private String froCss; @TableField("fro_vue") private String froVue; @TableField("fro_axios") private String froAxios; @TableField("fro_vuerouter") private String froVueRouter; @TableField("fro_vuex") private String froVuex; @TableField("fro_stuid") private Integer froStuid; @TableField("fro_del") private Integer froDel; }
这是一个名为Front的Java类,用于映射数据库表"front"的字段。该类使用了Lombok库的@Data注解,自动生成了getter和setter方法。
@TableField注解用于指定数据库表中对应的字段名。它可以在属性上使用,也可以在getter方法上使用。
@TableId注解用于指定表的主键字段,type = IdType.AUTO表示使用自增主键。
类中的属性对应了数据库表中的字段,包括froId、froHtml、froJs、froCss、froVue、froAxios、froVueRouter、froVuex、froStuid和froDel。
其中,froHtml、froJs、froCss、froVue、froAxios、froVueRouter和froVuex都是字符串类型的字段。
froStuid是一个整数类型的字段,用于表示与该前端相关联的学生ID。
froDel也是一个整数类型的字段,用于表示该前端是否被删除。
这个类的作用是将数据库表"front"中的数据映射到Java对象中,方便对数据进行操作。
springboot security项目 如何封装jwt 及token 其中数据表为package com.aokace.entity; import com.baomidou.mybatisplus.annotation.IdType; import com.baomidou.mybatisplus.annotation.TableField; import com.baomidou.mybatisplus.annotation.TableId; import com.baomidou.mybatisplus.annotation.TableName; import java.io.Serializable; import io.swagger.annotations.ApiModel; import io.swagger.annotations.ApiModelProperty; import lombok.Getter; import lombok.Setter; /** * <p> * * </p> * * @author aokace * @since 2023-05-23 */ @Getter @Setter @TableName("user") @ApiModel(value = "User对象", description = "") public class User implements Serializable { private static final long serialVersionUID = 1L; @TableId(value = "userid", type = IdType.AUTO) private Integer userid; @TableField("authority") private String authority; @TableField("role") private String role; @TableField("username") private String username; @TableField("password") private String password; }
首先需要引入相关的依赖,包括JWT和Spring Security的依赖:
```
<dependency>
<groupId>io.jsonwebtoken</groupId>
<artifactId>jjwt</artifactId>
<version>0.9.1</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
```
然后创建一个JwtTokenUtil类来实现JWT的签发和验证功能:
```java
import io.jsonwebtoken.Claims;
import io.jsonwebtoken.JwtBuilder;
import io.jsonwebtoken.Jwts;
import io.jsonwebtoken.SignatureAlgorithm;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.security.core.userdetails.UserDetails;
import org.springframework.stereotype.Component;
import java.util.Date;
import java.util.HashMap;
import java.util.Map;
@Component
public class JwtTokenUtil {
private static final String CLAIM_KEY_USERNAME = "sub";
private static final String CLAIM_KEY_CREATED = "created";
@Value("${jwt.secret}")
private String secret;
@Value("${jwt.expiration}")
private Long expiration;
public String generateToken(UserDetails userDetails) {
Map<String, Object> claims = new HashMap<>();
claims.put(CLAIM_KEY_USERNAME, userDetails.getUsername());
claims.put(CLAIM_KEY_CREATED, new Date());
return generateToken(claims);
}
public String getUsernameFromToken(String token) {
String username;
try {
Claims claims = getClaimsFromToken(token);
username = claims.getSubject();
} catch (Exception e) {
username = null;
}
return username;
}
public Date getCreatedDateFromToken(String token) {
Date created;
try {
Claims claims = getClaimsFromToken(token);
created = new Date((Long) claims.get(CLAIM_KEY_CREATED));
} catch (Exception e) {
created = null;
}
return created;
}
public Date getExpirationDateFromToken(String token) {
Date expiration;
try {
Claims claims = getClaimsFromToken(token);
expiration = claims.getExpiration();
} catch (Exception e) {
expiration = null;
}
return expiration;
}
public boolean isTokenExpired(String token) {
Date expiration = getExpirationDateFromToken(token);
return expiration.before(new Date());
}
public String refreshToken(String token) {
String refreshedToken;
try {
Claims claims = getClaimsFromToken(token);
claims.put(CLAIM_KEY_CREATED, new Date());
refreshedToken = generateToken(claims);
} catch (Exception e) {
refreshedToken = null;
}
return refreshedToken;
}
public boolean validateToken(String token, UserDetails userDetails) {
String username = getUsernameFromToken(token);
return username.equals(userDetails.getUsername()) && !isTokenExpired(token);
}
private Claims getClaimsFromToken(String token) {
Claims claims;
try {
claims = Jwts.parser()
.setSigningKey(secret)
.parseClaimsJws(token)
.getBody();
} catch (Exception e) {
claims = null;
}
return claims;
}
private String generateToken(Map<String, Object> claims) {
Date expirationDate = new Date(System.currentTimeMillis() + expiration * 1000);
JwtBuilder builder = Jwts.builder()
.setClaims(claims)
.setExpiration(expirationDate)
.signWith(SignatureAlgorithm.HS512, secret);
return builder.compact();
}
}
```
其中,JwtTokenUtil类中的generateToken方法用于生成JWT Token,getUsernameFromToken和getExpirationDateFromToken方法用于解析JWT Token中的用户名和过期时间,isTokenExpired方法用于判断JWT Token是否已经过期,refreshToken方法用于刷新JWT Token,validateToken方法用于验证JWT Token是否有效,getClaimsFromToken方法用于从JWT Token中获取Claims。
然后在Spring Security的配置类中添加JwtTokenFilter来实现JWT的过滤和验证:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.authentication.AuthenticationManager;
import org.springframework.security.authentication.UsernamePasswordAuthenticationToken;
import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder;
import org.springframework.security.config.annotation.method.configuration.EnableGlobalMethodSecurity;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
import org.springframework.security.config.http.SessionCreationPolicy;
import org.springframework.security.core.Authentication;
import org.springframework.security.core.AuthenticationException;
import org.springframework.security.core.userdetails.UserDetailsService;
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
import org.springframework.security.web.authentication.UsernamePasswordAuthenticationFilter;
@Configuration
@EnableWebSecurity
@EnableGlobalMethodSecurity(prePostEnabled = true)
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private UserDetailsService userDetailsService;
@Autowired
private JwtTokenFilter jwtTokenFilter;
@Autowired
private JwtAuthenticationEntryPoint jwtAuthenticationEntryPoint;
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(userDetailsService).passwordEncoder(new BCryptPasswordEncoder());
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf().disable()
.authorizeRequests()
.antMatchers("/login").permitAll()
.anyRequest().authenticated()
.and()
.exceptionHandling().authenticationEntryPoint(jwtAuthenticationEntryPoint)
.and()
.sessionManagement().sessionCreationPolicy(SessionCreationPolicy.STATELESS);
http.addFilterBefore(jwtTokenFilter, UsernamePasswordAuthenticationFilter.class);
}
@Override
public AuthenticationManager authenticationManagerBean() throws Exception {
return super.authenticationManagerBean();
}
}
```
其中,SecurityConfig类中的configure方法用于配置Spring Security的策略,addFilterBefore方法用于添加JwtTokenFilter,authenticationManagerBean方法用于获取AuthenticationManager。
最后,在登录接口中,使用JwtTokenUtil生成JWT Token并返回给前端:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.ResponseEntity;
import org.springframework.security.authentication.AuthenticationManager;
import org.springframework.security.authentication.UsernamePasswordAuthenticationToken;
import org.springframework.security.core.Authentication;
import org.springframework.security.core.userdetails.UserDetails;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class AuthController {
@Autowired
private AuthenticationManager authenticationManager;
@Autowired
private JwtTokenUtil jwtTokenUtil;
@Autowired
private UserDetailsService userDetailsService;
@PostMapping("/login")
public ResponseEntity<?> login(@RequestBody AuthRequest authRequest) throws Exception {
try {
Authentication authentication = authenticationManager.authenticate(
new UsernamePasswordAuthenticationToken(authRequest.getUsername(), authRequest.getPassword())
);
UserDetails userDetails = userDetailsService.loadUserByUsername(authRequest.getUsername());
String token = jwtTokenUtil.generateToken(userDetails);
return ResponseEntity.ok(new AuthResponse(token));
} catch (Exception e) {
throw new Exception("Incorrect username or password", e);
}
}
}
```
其中,AuthController类中的login方法用于处理登录请求,通过authenticationManager.authenticate方法验证用户名和密码,然后使用JwtTokenUtil生成JWT Token并返回给前端。
阅读全文
相关推荐
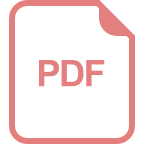
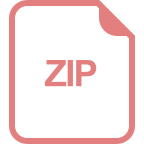
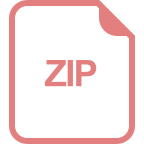











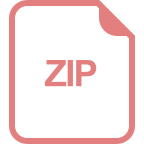
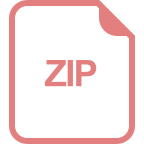
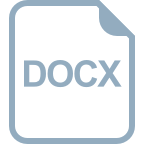