改进该段代码#include <iostream> #include <winsock2.h> #include <iphlpapi.h> #include <icmpapi.h> using namespace std; #pragma comment(lib, "iphlpapi.lib") #pragma comment(lib, "ws2_32.lib") int main(int argc, char* argv[]) { WSADATA wsaData; int iResult = WSAStartup(MAKEWORD(2, 2), &wsaData); if (iResult != 0) { cout << "WSAStartup failed: " << iResult << std::endl; return 1; } if (argc < 2) { cout << "Usage: ping <hostname or IP address>" << std::endl; return 1; } string host = argv[1]; HANDLE hIcmpFile = IcmpCreateFile(); if (hIcmpFile == INVALID_HANDLE_VALUE) { cout << "IcmpCreateFile failed: " << GetLastError() << std::endl; WSACleanup(); return 1; } char SendData[] = "Ping"; LPVOID ReplyBuffer = malloc(sizeof(ICMP_ECHO_REPLY) + sizeof(SendData)); if (ReplyBuffer == NULL) { cout << "malloc failed: " << GetLastError() << std::endl; IcmpCloseHandle(hIcmpFile); WSACleanup(); return 1; } DWORD ReplySize = sizeof(ICMP_ECHO_REPLY) + sizeof(SendData); DWORD dwRetVal = IcmpSendEcho(hIcmpFile, inet_addr(host.c_str()), SendData, sizeof(SendData), NULL, ReplyBuffer, ReplySize, 1000); if (dwRetVal != 0) { PICMP_ECHO_REPLY pEchoReply = (PICMP_ECHO_REPLY)ReplyBuffer; cout << "Ping " << host << " successful: " << "Reply from " << inet_ntoa(pEchoReply->Address) << " time=" << pEchoReply->RoundTripTime << "ms" << std::endl; } else { cout << "Ping " << host << " failed: " << GetLastError() << std::endl; } free(ReplyBuffer); IcmpCloseHandle(hIcmpFile); WSACleanup(); return 0; }
时间: 2024-01-22 09:01:55 浏览: 168
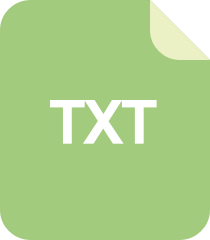
vc++2008编译不了#include<iostream.h>头文件

这段代码是一个简单的ping程序,但是可以进行一些改进:
1. 错误处理:在程序中应该对所有可能出现的错误进行处理,例如WSAStartup、IcmpCreateFile、malloc、IcmpSendEcho等函数返回值不为0时应该输出错误信息并退出程序。
2. 参数处理:如果参数不正确,应该输出正确的用法并退出程序。
3. 变量命名:变量名应该具有一定的描述性,例如ReplyBuffer可以改为pReplyBuffer,dwRetVal可以改为dwEchoReply。
改进后的代码如下所示:
```c
#include <iostream>
#include <winsock2.h>
#include <iphlpapi.h>
#include <icmpapi.h>
using namespace std;
#pragma comment(lib, "iphlpapi.lib")
#pragma comment(lib, "ws2_32.lib")
int main(int argc, char* argv[]) {
WSADATA wsaData;
int iResult = WSAStartup(MAKEWORD(2, 2), &wsaData);
if (iResult != 0) {
cout << "WSAStartup failed: " << iResult << endl;
return 1;
}
if (argc != 2) {
cout << "Usage: ping <hostname or IP address>" << endl;
return 1;
}
string host = argv[1];
HANDLE hIcmpFile = IcmpCreateFile();
if (hIcmpFile == INVALID_HANDLE_VALUE) {
cout << "IcmpCreateFile failed: " << GetLastError() << endl;
WSACleanup();
return 1;
}
char SendData[] = "Ping";
DWORD dwReplyBufferSize = sizeof(ICMP_ECHO_REPLY) + sizeof(SendData);
LPVOID pReplyBuffer = malloc(dwReplyBufferSize);
if (pReplyBuffer == NULL) {
cout << "malloc failed: " << GetLastError() << endl;
IcmpCloseHandle(hIcmpFile);
WSACleanup();
return 1;
}
DWORD dwEchoReply = IcmpSendEcho(hIcmpFile, inet_addr(host.c_str()), SendData, sizeof(SendData), NULL, pReplyBuffer, dwReplyBufferSize, 1000);
if (dwEchoReply == 0) {
cout << "Ping " << host << " failed: " << GetLastError() << endl;
free(pReplyBuffer);
IcmpCloseHandle(hIcmpFile);
WSACleanup();
return 1;
}
PICMP_ECHO_REPLY pEchoReply = (PICMP_ECHO_REPLY)pReplyBuffer;
cout << "Ping " << host << " successful: " << "Reply from " << inet_ntoa(pEchoReply->Address) << " time=" << pEchoReply->RoundTripTime << "ms" << endl;
free(pReplyBuffer);
IcmpCloseHandle(hIcmpFile);
WSACleanup();
return 0;
}
```
改进后的代码加入了错误处理和参数处理,变量名也更加具有描述性,可以更加安全和方便地使用。
阅读全文
相关推荐
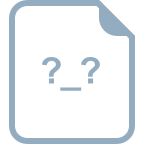



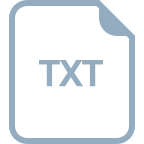
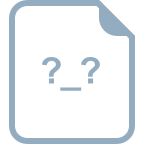
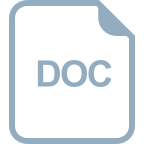
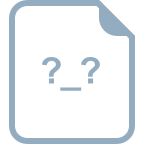
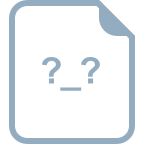
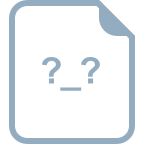
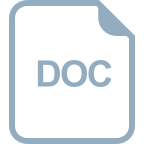
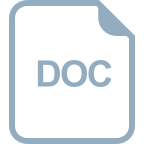
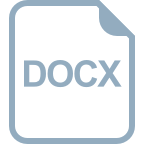
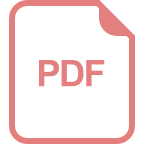
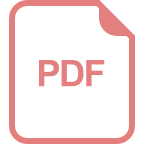
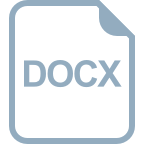
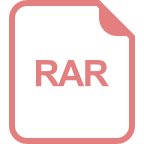