用c语言实现输入n个字符建立单链表,删除表中重复元素并修改计数,实现单链表的统计
时间: 2023-05-20 15:03:33 浏览: 132
非常感谢您的问题!以下是用 C 语言实现输入 n 个字符建立单链表,删除表中重复元素并修改计数,实现单链表的统计的代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct node {
char data;
int count;
struct node *next;
};
struct node *create_list(char *str, int n) {
struct node *head, *p, *q;
head = (struct node *)malloc(sizeof(struct node));
head->next = NULL;
q = head;
for (int i = 0; i < n; i++) {
p = (struct node *)malloc(sizeof(struct node));
p->data = str[i];
p->count = 1;
p->next = NULL;
q->next = p;
q = p;
}
return head;
}
void delete_duplicate(struct node *head) {
struct node *p, *q, *r;
p = head->next;
while (p != NULL) {
q = p->next;
r = p;
while (q != NULL) {
if (q->data == p->data) {
r->next = q->next;
p->count++;
free(q);
q = r->next;
} else {
r = q;
q = q->next;
}
}
p = p->next;
}
}
void print_list(struct node *head) {
struct node *p;
p = head->next;
while (p != NULL) {
printf("%c:%d ", p->data, p->count);
p = p->next;
}
printf("\n");
}
int main() {
char str[100];
int n;
printf("请输入字符串长度n:");
scanf("%d", &n);
printf("请输入字符串:");
scanf("%s", str);
struct node *head = create_list(str, n);
delete_duplicate(head);
print_list(head);
return 0;
}
```
希望这个代码能够帮到您!
阅读全文
相关推荐
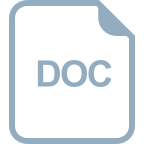
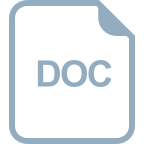
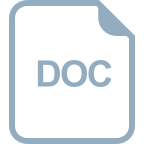
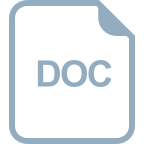
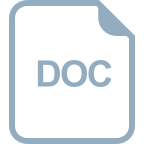
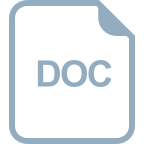
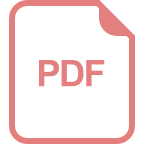
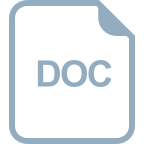


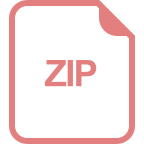
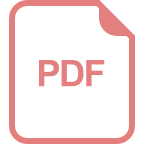
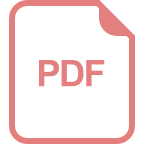
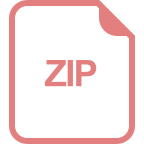
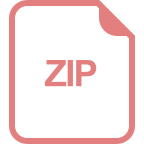
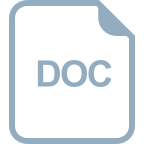
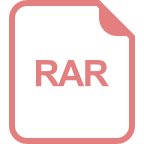
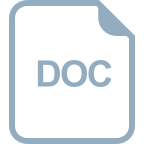
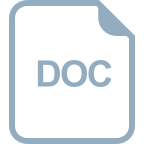