数据结构线性表查找代码
时间: 2023-07-06 22:33:58 浏览: 76
以下是一个简单的线性表查找代码示例:
```C++
#include <iostream>
using namespace std;
const int MAX_SIZE = 100; // 线性表最大长度
struct List {
int data[MAX_SIZE]; // 存储元素的数组
int length; // 线性表当前长度
};
// 初始化一个空的线性表
void initList(List &L) {
L.length = 0;
}
// 在线性表的末尾插入一个元素
void insert(List &L, int x) {
if (L.length >= MAX_SIZE) {
cout << "Error: Linear list is full!" << endl;
return;
}
L.data[L.length] = x;
L.length++;
}
// 在线性表中查找值为x的元素,返回其下标,如果找不到则返回-1
int search(List L, int x) {
for (int i = 0; i < L.length; i++) {
if (L.data[i] == x) {
return i;
}
}
return -1;
}
int main() {
List L;
initList(L);
insert(L, 1);
insert(L, 2);
insert(L, 3);
int x;
cout << "Please enter the value you want to search: ";
cin >> x;
int index = search(L, x);
if (index == -1) {
cout << "The value " << x << " is not found in the linear list." << endl;
} else {
cout << "The value " << x << " is found at index " << index << " in the linear list." << endl;
}
return 0;
}
```
在该代码中,我们使用结构体List来表示线性表,其中包含一个数组data和一个长度length。我们提供了initList和insert函数,使得我们可以初始化一个空的线性表并在末尾插入元素。search函数则用于在线性表中查找指定值的元素。
相关推荐
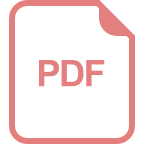
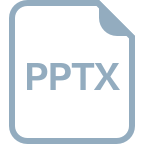
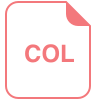
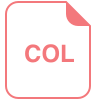
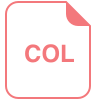
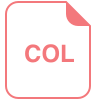
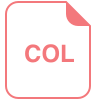









