给定一个M×N的迷宫图、入口与出口、行走规则。求一条从指定入口到 出口的路径。所求路径必须是简单路径,即路径不重复。使用栈的结构完成。要求用c语言
时间: 2024-03-28 16:41:23 浏览: 26
好的,我可以帮您解答这个问题。
首先,我们需要定义一个迷宫的结构体,用于存储迷宫的信息:
```c
#define MAX_ROW 100
#define MAX_COL 100
typedef struct {
int row;
int col;
int dir;
} element;
typedef struct {
int maze[MAX_ROW][MAX_COL];
int row, col;
element entry, exit;
} maze;
```
`maze` 结构体中,`maze` 数组存储了迷宫的信息,`entry` 和 `exit` 分别表示入口和出口的位置,`row` 和 `col` 分别表示迷宫的行数和列数。
接下来,我们需要定义一个栈的结构体,用于存储路径上的元素:
```c
#define MAX_STACK_SIZE 10000
typedef struct {
element data[MAX_STACK_SIZE];
int top;
} StackType;
```
`StackType` 结构体中,`data` 数组用于存储路径上的元素,`top` 表示栈顶元素的位置。
现在,我们可以开始编写求解迷宫路径的函数了。核心代码如下:
```c
void path(maze *m)
{
int i, row, col, next_row, next_col, dir;
element position;
StackType s;
s.top = -1;
m->maze[m->entry.row][m->entry.col] = 2; // 标记入口
position = m->entry; // 入口作为起点
push(&s, position); // 将起点入栈
while (s.top > -1) {
position = pop(&s); // 弹出栈顶元素
row = position.row;
col = position.col;
dir = position.dir;
while (dir < 4) { // 枚举下一个位置
next_row = row + move[dir].vert;
next_col = col + move[dir].horiz;
if (next_row == m->exit.row && next_col == m->exit.col) { // 到达出口
push(&s, position); // 将当前位置入栈
position.row = next_row;
position.col = next_col;
position.dir = dir;
push(&s, position); // 将出口入栈
print_stack(&s); // 输出路径
return;
} else if (m->maze[next_row][next_col] == 0) { // 可以走
position.dir = ++dir;
push(&s, position); // 将当前位置入栈
row = next_row;
col = next_col;
dir = 0;
position.row = row;
position.col = col;
position.dir = dir;
push(&s, position); // 将下一位置入栈
m->maze[row][col] = 2; // 标记已走过
} else {
++dir; // 不能走,尝试下一个方向
}
}
}
printf("找不到出口!\n");
}
```
这个函数使用了深度优先搜索算法,在栈的帮助下完成了路径的搜索。具体实现过程如下:
1. 初始化栈,将入口入栈,并标记入口已走过。
2. 不断从栈中弹出元素,直到栈为空。
3. 对于弹出的元素,枚举下一个可能的位置,并判断该位置是否为出口:
- 如果到达出口,则将当前位置和出口都入栈,并输出路径;
- 如果可以走,则将当前位置和下一个位置都入栈,并标记下一个位置已走过;
- 如果不能走,则尝试下一个方向。
4. 如果栈为空,说明找不到出口。
相关推荐
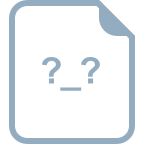





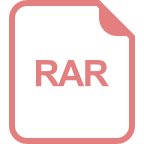
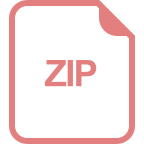
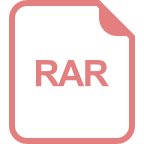
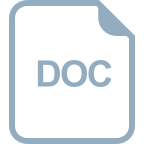
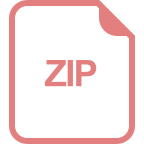
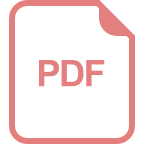
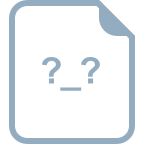
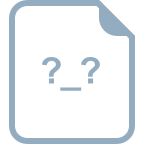