vue+springboot做一个价格日历,前后端代码
时间: 2024-01-15 16:02:44 浏览: 98
因为价格日历需要与数据库进行交互,并且涉及到前后端交互,所以需要编写前后端代码。
1. 前端代码
使用Vue.js框架编写前端代码,主要包括以下几个部分:
(1)页面布局:使用HTML和CSS编写价格日历的布局,可以使用element-ui等UI框架进行美化。
(2)数据绑定:使用Vue.js的双向数据绑定功能将前端页面与后端数据进行交互。
(3)事件处理:为价格日历添加事件处理方法,如点击日期时弹出价格详情等。
(4)AJAX请求:使用axios等工具向后端发送请求获取数据,并将数据渲染到页面上。
示例代码:
```vue
<template>
<div>
<el-calendar v-model="date" :range="range" :first-day-of-week="1" :cell-style="cellStyle" @change="handleDateChange"></el-calendar>
<el-dialog title="价格详情" :visible.sync="dialogVisible">
<p>日期:{{ selectedDate }}</p>
<p>价格:{{ price }}</p>
</el-dialog>
</div>
</template>
<script>
import axios from 'axios'
export default {
data() {
return {
date: null,
range: [new Date(2020, 0, 1), new Date(2021, 11, 31)],
dialogVisible: false,
selectedDate: '',
price: ''
}
},
methods: {
cellStyle({ date, row, column }) {
return {
backgroundColor: 'white'
}
},
handleDateChange() {
const year = this.date.getFullYear()
const month = this.date.getMonth() + 1
const day = this.date.getDate()
axios.get(`/api/price?date=${year}-${month}-${day}`).then(res => {
this.price = res.data.price
}).catch(() => {
this.price = '无价格数据'
})
this.selectedDate = `${year}-${month}-${day}`
this.dialogVisible = true
}
}
}
</script>
```
2. 后端代码
使用Spring Boot框架编写后端代码,主要包括以下几个部分:
(1)数据模型:定义价格数据模型,包括日期和价格两个字段。
(2)数据访问:使用JPA等工具访问数据库,实现对价格数据的增删改查等功能。
(3)控制器:编写RESTful API控制器,接收前端发送的请求,并根据请求参数返回对应的数据。
示例代码:
```java
@Entity
public class Price {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private LocalDate date;
private double price;
// getters and setters
}
@Repository
public interface PriceRepository extends JpaRepository<Price, Long> {
Optional<Price> findByDate(LocalDate date);
}
@RestController
public class PriceController {
@Autowired
private PriceRepository priceRepository;
@GetMapping("/api/price")
public ResponseEntity<?> getPriceByDate(@RequestParam("date") @DateTimeFormat(iso = DateTimeFormat.ISO.DATE) LocalDate date) {
Optional<Price> price = priceRepository.findByDate(date);
if (price.isPresent()) {
return ResponseEntity.ok(price.get());
} else {
return ResponseEntity.notFound().build();
}
}
}
```
阅读全文
相关推荐
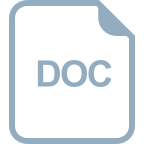
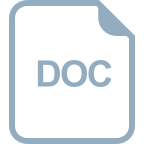
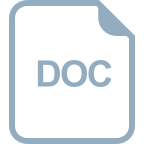
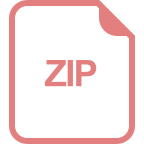
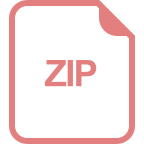
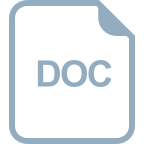
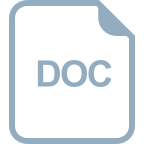
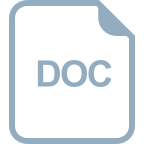


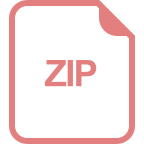
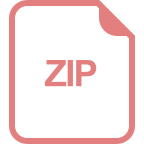
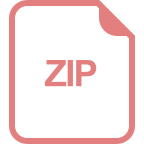
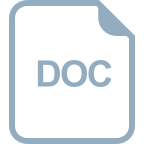
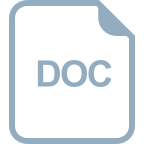
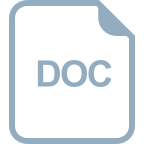
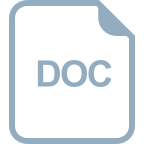
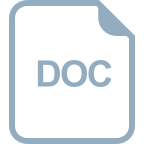
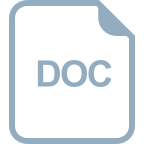
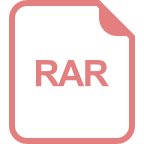