帮我写一个能实现大数相加并将参数传至private的类,不使用stringbuilder
时间: 2023-05-26 12:02:13 浏览: 117
这是一个实现大数相加,并将参数传递至 private 属性的类示例。
```
public class LargeNumberAdder {
// 使用数组来存储大数值
private int[] num1;
private int[] num2;
// 构造函数,用于初始化 num1 和 num2 数组
public LargeNumberAdder(int[] num1, int[] num2) {
this.num1 = num1;
this.num2 = num2;
}
// 实现大数相加功能并返回结果
public int[] add() {
int maxLength = Math.max(num1.length, num2.length);
int[] result = new int[maxLength];
int carry = 0;
for (int i = 0; i < maxLength; i++) {
int sum = carry;
if (i < num1.length) {
sum += num1[num1.length - 1 - i];
}
if (i < num2.length) {
sum += num2[num2.length - 1 - i];
}
result[maxLength - 1 - i] = sum % 10;
carry = sum / 10;
}
// 如果最高位有进位,则需要扩充数组
if (carry > 0) {
int[] newResult = new int[maxLength + 1];
System.arraycopy(result, 0, newResult, 1, maxLength);
newResult[0] = carry;
return newResult;
}
return result;
}
}
```
使用方法:
```
int[] num1 = { 9, 9, 9, 9 };
int[] num2 = { 1, 0, 0, 0 };
LargeNumberAdder adder = new LargeNumberAdder(num1, num2);
int[] result = adder.add();
// 输出结果
for (int i : result) {
System.out.print(i);
}
```
阅读全文
相关推荐
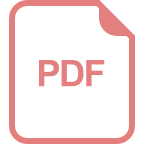
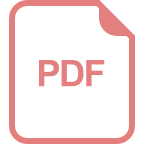
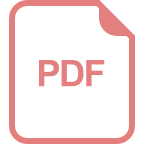















