用python开发一个在线投票系统,实现添加投票候选人,删除候选人,为候选人投票,按序号投票,删除投票,清空投票,投票统计,退出投票
时间: 2024-02-15 22:07:40 浏览: 197
好的,这是一个比较复杂的项目,需要设计一些数据结构和算法来实现。以下是一种可能的实现方式,供参考:
首先,我们需要一个字典来存储候选人和他们的得票数,以候选人的名字为键,得票数为值:
```python
candidates = {}
```
然后,我们可以定义一些函数来实现各种功能:
1. 添加候选人
```python
def add_candidate(name):
if name not in candidates:
candidates[name] = 0
print("候选人 %s 添加成功!" % name)
else:
print("候选人 %s 已存在!" % name)
```
2. 删除候选人
```python
def delete_candidate(name):
if name in candidates:
del candidates[name]
print("候选人 %s 删除成功!" % name)
else:
print("候选人 %s 不存在!" % name)
```
3. 为候选人投票
```python
def vote_for_candidate(name):
if name in candidates:
candidates[name] += 1
print("候选人 %s 得票数加1,当前得票数为 %d。" % (name, candidates[name]))
else:
print("候选人 %s 不存在!" % name)
```
4. 按序号投票
```python
def vote_by_index(index):
if index >= 1 and index <= len(candidates):
name = list(candidates.keys())[index - 1]
candidates[name] += 1
print("候选人 %s 得票数加1,当前得票数为 %d。" % (name, candidates[name]))
else:
print("序号 %d 不合法!" % index)
```
5. 删除投票
```python
def delete_vote(name):
if name in candidates:
candidates[name] -= 1
print("候选人 %s 得票数减1,当前得票数为 %d。" % (name, candidates[name]))
else:
print("候选人 %s 不存在!" % name)
```
6. 清空投票
```python
def clear_votes():
candidates.clear()
print("投票数据已清空!")
```
7. 投票统计
```python
def show_results():
if len(candidates) == 0:
print("当前没有候选人!")
else:
print("投票结果如下:")
for name in candidates:
print("%s: %d" % (name, candidates[name]))
```
8. 退出投票
```python
def exit_vote():
print("感谢您的使用,再见!")
exit()
```
以上是一个简单的在线投票系统的实现,你可以根据自己的需求进行修改和完善。
阅读全文
相关推荐
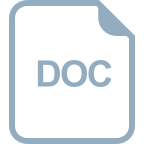
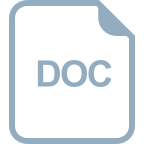
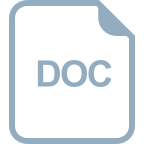







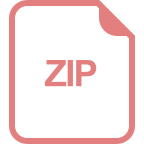








