切比雪夫低通滤波器 c语言实现
时间: 2023-07-13 07:16:02 浏览: 249
以下是一个简单的C语言实现切比雪夫低通滤波器的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define PI 3.14159265358979323846
double chebyshev(int n, double x) {
if (n == 0) {
return 1;
} else if (n == 1) {
return x;
} else {
return 2 * x * chebyshev(n-1, x) - chebyshev(n-2, x);
}
}
void chebyshev_lpfilter(double *h, int n, double fc, double epsilon) {
int i;
double x, u, a, b, c, d;
for (i = 0; i <= n; i++) {
x = cos(PI * i / n);
u = sinh(asinh(1/epsilon) / n);
a = 1;
b = -2 * x;
c = 1 + u * u;
d = -2 * u * x;
h[i] = 1 / (a + b + c) * (a * (c + b) + d);
}
}
int main() {
int i, n = 10;
double fc = 0.3, epsilon = 0.5;
double *h = (double *)calloc(n+1, sizeof(double));
chebyshev_lpfilter(h, n, fc, epsilon);
for (i = 0; i <= n; i++) {
printf("%f ", h[i]);
}
printf("\n");
free(h);
return 0;
}
```
该代码中实现了一个10阶的切比雪夫低通滤波器,其截止频率为0.3,通带波纹系数为0.5。其中,`chebyshev()`函数用于计算切比雪夫多项式,`chebyshev_lpfilter()`函数用于计算切比雪夫低通滤波器的FIR系数。在`main()`函数中,先调用`chebyshev_lpfilter()`函数计算FIR系数,然后输出结果。
阅读全文
相关推荐
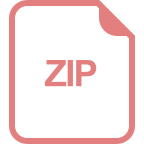
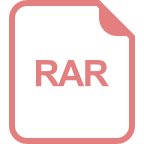
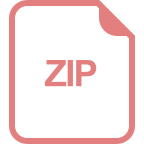















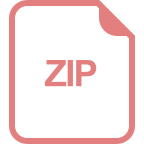