写一个c++ 遍历文件夹的代码
时间: 2023-09-26 18:07:00 浏览: 71
### 回答1:
#include <stdio.h>
#include <dirent.h>int main(int argc, char *argv[])
{
DIR *dir;
struct dirent *entry; if ((dir = opendir(argv[1])) == NULL) {
printf("Error al abrir el directorio.\n");
return 1;
} while ((entry = readdir(dir)) != NULL) {
printf("%s\n", entry->d_name);
} closedir(dir);
return 0;
}
### 回答2:
以下是一个使用C语言编写的遍历文件夹的代码:
```c
#include <stdio.h>
#include <dirent.h>
#include <string.h>
void traverseFolder(const char *folderPath) {
DIR *dir;
struct dirent *entry;
// 打开目标文件夹
dir = opendir(folderPath);
if (dir == NULL) {
printf("无法打开文件夹\n");
return;
}
// 读取目标文件夹中的每个文件或子文件夹
while ((entry = readdir(dir)) != NULL) {
if (strcmp(entry->d_name, ".") != 0 && strcmp(entry->d_name, "..") != 0) {
// 判断是否是文件夹
if (entry->d_type == DT_DIR) {
char path[256];
snprintf(path, sizeof(path), "%s/%s", folderPath, entry->d_name);
printf("文件夹:%s\n", path);
traverseFolder(path); // 递归调用函数遍历子文件夹
} else {
char path[256];
snprintf(path, sizeof(path), "%s/%s", folderPath, entry->d_name);
printf("文件:%s\n", path);
}
}
}
// 关闭目标文件夹
closedir(dir);
}
int main() {
const char *folderPath = "/path/to/folder"; // 替换为目标文件夹的路径
traverseFolder(folderPath);
return 0;
}
```
该代码首先通过调用`opendir`函数打开目标文件夹,然后使用`readdir`函数逐个读取目录中的文件和子文件夹。然后通过判断`d_type`字段的值,可以确定当前项是文件夹还是文件。如果是文件夹,则使用递归方式调用`traverseFolder`函数遍历该子文件夹。如果是文件,则直接输出文件路径。最后使用`closedir`函数关闭目标文件夹。将`/path/to/folder`替换为实际的目标文件夹路径即可进行测试。
### 回答3:
下面是一个用 C 语言编写的遍历文件夹的代码:
```c
#include <stdio.h>
#include <dirent.h>
#include <string.h>
// 递归遍历文件夹
void traverseDir(const char *dir) {
DIR *dp;
struct dirent *entry;
// 打开目录
dp = opendir(dir);
if (dp == NULL) {
printf("无法打开目录。\n");
return;
}
// 读取目录中的条目
while ((entry = readdir(dp)) != NULL) {
// 忽略 "." 和 ".." 目录
if (strcmp(entry->d_name, ".") == 0 || strcmp(entry->d_name, "..") == 0)
continue;
// 拼接文件路径
char path[256];
sprintf(path, "%s/%s", dir, entry->d_name);
// 判断是否为目录
if (entry->d_type == DT_DIR) {
// 是目录,递归遍历
traverseDir(path);
} else {
// 不是目录,打印文件名
printf("%s\n", path);
}
}
// 关闭目录
closedir(dp);
}
int main() {
const char *dir = "/path/to/folder"; // 替换为实际的文件夹路径
// 调用遍历函数
traverseDir(dir);
return 0;
}
```
以上代码使用递归的方式遍历指定文件夹下的所有文件和子文件夹,并将文件路径打印出来。请将代码中的 `"/path/to/folder"` 替换为实际的文件夹路径。
相关推荐
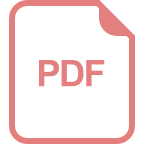
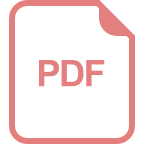














