c语言编程使用消息队列从父进程发送10个整数到子进程,子进程收下来后计算总和,后用同一个消息队列把总和发回给父进程显示
时间: 2023-05-29 20:04:48 浏览: 165
父进程代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#define MSG_KEY 1234
struct msgbuf {
long mtype;
int mtext;
};
int main() {
int i;
int sum = 0;
struct msgbuf msg;
// 创建消息队列
int msgid = msgget(MSG_KEY, IPC_CREAT | 0666);
if (msgid == -1) {
perror("msgget failed");
exit(EXIT_FAILURE);
}
// 发送消息给子进程
for (i = 1; i <= 10; i++) {
msg.mtype = 1;
msg.mtext = i;
if (msgsnd(msgid, &msg, sizeof(msg.mtext), 0) == -1) {
perror("msgsnd failed");
exit(EXIT_FAILURE);
}
}
// 接收子进程发送的消息
if (msgrcv(msgid, &msg, sizeof(msg.mtext), 2, 0) == -1) {
perror("msgrcv failed");
exit(EXIT_FAILURE);
}
sum = msg.mtext;
printf("Sum is %d\n", sum);
// 删除消息队列
if (msgctl(msgid, IPC_RMID, NULL) == -1) {
perror("msgctl failed");
exit(EXIT_FAILURE);
}
return 0;
}
```
子进程代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#define MSG_KEY 1234
struct msgbuf {
long mtype;
int mtext;
};
int main() {
int i;
int sum = 0;
struct msgbuf msg;
// 创建消息队列
int msgid = msgget(MSG_KEY, 0666);
if (msgid == -1) {
perror("msgget failed");
exit(EXIT_FAILURE);
}
// 接收父进程发送的消息
for (i = 1; i <= 10; i++) {
if (msgrcv(msgid, &msg, sizeof(msg.mtext), 1, 0) == -1) {
perror("msgrcv failed");
exit(EXIT_FAILURE);
}
sum += msg.mtext;
}
// 发送总和给父进程
msg.mtype = 2;
msg.mtext = sum;
if (msgsnd(msgid, &msg, sizeof(msg.mtext), 0) == -1) {
perror("msgsnd failed");
exit(EXIT_FAILURE);
}
return 0;
}
```
阅读全文
相关推荐
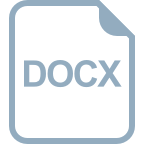


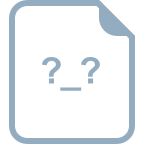
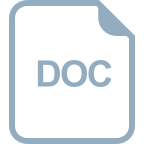
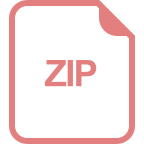
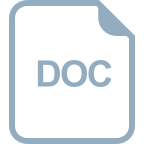
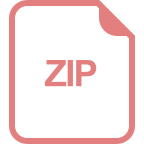
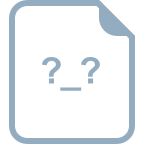
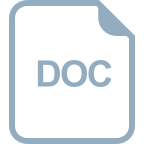
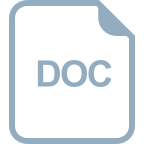
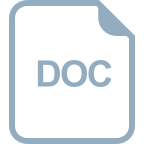
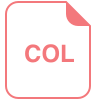