java设计矩阵类matrix代码
时间: 2023-10-30 21:08:46 浏览: 241
好的,下面是一份简单的 Java 矩阵类 Matrix 的代码:
```java
public class Matrix {
private final int rows;
private final int cols;
private final double[][] data;
public Matrix(int rows, int cols) {
if (rows <= 0 || cols <= 0) {
throw new IllegalArgumentException("rows and cols must be positive");
}
this.rows = rows;
this.cols = cols;
this.data = new double[rows][cols];
}
public int getRows() {
return rows;
}
public int getCols() {
return cols;
}
public double get(int i, int j) {
checkBounds(i, j);
return data[i][j];
}
public void set(int i, int j, double x) {
checkBounds(i, j);
data[i][j] = x;
}
private void checkBounds(int i, int j) {
if (i < 0 || i >= rows) {
throw new IndexOutOfBoundsException("row index out of bounds");
}
if (j < 0 || j >= cols) {
throw new IndexOutOfBoundsException("col index out of bounds");
}
}
public static Matrix add(Matrix a, Matrix b) {
if (a.rows != b.rows || a.cols != b.cols) {
throw new IllegalArgumentException("matrices must have the same dimensions");
}
Matrix c = new Matrix(a.rows, a.cols);
for (int i = 0; i < a.rows; i++) {
for (int j = 0; j < a.cols; j++) {
c.data[i][j] = a.data[i][j] + b.data[i][j];
}
}
return c;
}
public static Matrix multiply(Matrix a, Matrix b) {
if (a.cols != b.rows) {
throw new IllegalArgumentException("number of columns in A must match number of rows in B");
}
Matrix c = new Matrix(a.rows, b.cols);
for (int i = 0; i < a.rows; i++) {
for (int j = 0; j < b.cols; j++) {
double sum = 0.0;
for (int k = 0; k < a.cols; k++) {
sum += a.data[i][k] * b.data[k][j];
}
c.data[i][j] = sum;
}
}
return c;
}
}
```
这个矩阵类支持以下操作:
- 创建矩阵对象:`Matrix m = new Matrix(rows, cols)`
- 获取矩阵的行数和列数:`m.getRows()` 和 `m.getCols()`
- 获取矩阵的某个元素:`m.get(i, j)`
- 设置矩阵的某个元素:`m.set(i, j, x)`
- 矩阵加法:`Matrix.add(a, b)`
- 矩阵乘法:`Matrix.multiply(a, b)`
阅读全文
相关推荐
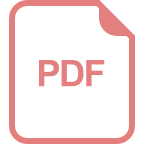




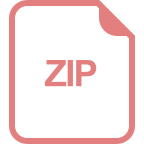
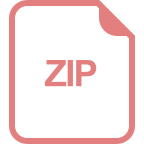
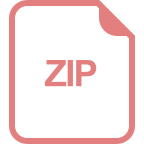
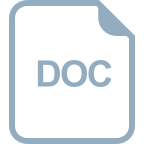





