写一段可以生成词云的代码
时间: 2024-05-14 13:14:34 浏览: 69
以下是一个可以生成词云的Python代码示例:
```python
# 导入所需的库
from wordcloud import WordCloud
import matplotlib.pyplot as plt
# 读取文本文件
with open('text.txt', 'r', encoding='utf-8') as f:
text = f.read()
# 创建WordCloud对象
wordcloud = WordCloud(background_color='white', font_path='msyh.ttc', width=800, height=600)
# 生成词云
wordcloud.generate(text)
# 显示词云
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
plt.show()
# 保存词云图片
wordcloud.to_file('wordcloud.png')
```
在上述代码中,我们首先使用`open()`函数读取文本文件,然后使用`WordCloud`类创建一个词云对象。通过调用`generate()`方法,我们可以将文本文件中的内容转换为词云。最后,我们使用`imshow()`函数将词云显示在屏幕上,并使用`to_file()`方法将词云保存为PNG格式。其中,`background_color`参数指定词云的背景颜色,`font_path`参数指定字体文件路径,`width`和`height`参数指定词云的宽度和高度。
相关问题
帮我写一段生成词云图的代码
好的,以下是一段使用Python生成词云图的代码,需要使用wordcloud库和matplotlib库:
```python
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
from wordcloud import WordCloud, STOPWORDS
# 读取数据
data = pd.read_csv('data.csv', encoding='utf-8')
# 去除停用词
stopwords = set(STOPWORDS)
words = []
for i in data['text']:
for j in i.split():
if j not in stopwords:
words.append(j)
# 生成词云图
wordcloud = WordCloud(width=800, height=800, background_color='white', min_font_size=10).generate(' '.join(words))
# 显示词云图
plt.figure(figsize=(8, 8), facecolor=None)
plt.imshow(wordcloud)
plt.axis("off")
plt.tight_layout(pad=0)
plt.show()
```
其中,`data.csv`是需要生成词云图的数据文件,`text`是需要生成词云图的文本数据所在的列名。在生成词云图之前,需要先去除停用词,以保证生成的词云图更加精准和有意义。
用Python写一个可以生成词云图的小程序的代码
好的,以下是可以生成词云图的Python代码:
```python
import numpy as np
import matplotlib.pyplot as plt
from wordcloud import WordCloud
# 读取文本文件
with open('text.txt', 'r', encoding='utf-8') as f:
text = f.read()
# 生成词云
wordcloud = WordCloud(background_color='white', width=800, height=600, margin=2).generate(text)
# 显示词云
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
plt.show()
```
用此代码,你可以生成一个名为 `text.txt` 的文本文件,并在其中写入文本内容。然后,运行这段代码,就会生成一个词云图并显示出来。你也可以根据自己的需要调整一些参数(例如背景颜色、宽高等)。
阅读全文
相关推荐
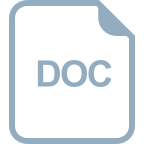
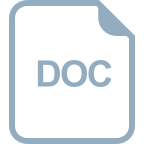
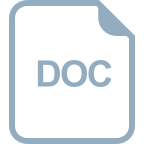
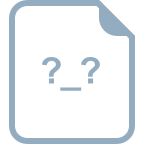
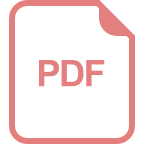











