qmap<qstring,int>比较不同的键和值是否一样
时间: 2024-02-01 11:13:54 浏览: 27
可以使用两个迭代器遍历两个qmap对象,比较它们的键和值是否相同。示例代码如下:
```c++
bool isEqual(const QMap<QString, int>& map1, const QMap<QString, int>& map2)
{
// 如果两个map的大小不一样,直接返回false
if (map1.size() != map2.size()) {
return false;
}
// 遍历map1,查找对应的键和值是否在map2中也存在
for (auto i = map1.constBegin(); i != map1.constEnd(); ++i) {
auto j = map2.constFind(i.key());
// 如果map2中不存在当前键,或者对应的值不相等,返回false
if (j == map2.constEnd() || *j != i.value()) {
return false;
}
}
// 遍历map2,查找对应的键和值是否在map1中也存在
for (auto i = map2.constBegin(); i != map2.constEnd(); ++i) {
auto j = map1.constFind(i.key());
// 如果map1中不存在当前键,或者对应的值不相等,返回false
if (j == map1.constEnd() || *j != i.value()) {
return false;
}
}
// 如果所有的键和值都相等,返回true
return true;
}
```
调用方式:
```c++
QMap<QString, int> map1{{"a", 1}, {"b", 2}, {"c", 3}};
QMap<QString, int> map2{{"a", 1}, {"b", 2}, {"c", 3}};
QMap<QString, int> map3{{"a", 1}, {"b", 2}, {"c", 4}};
if (isEqual(map1, map2)) {
qDebug() << "map1 and map2 are equal.";
} else {
qDebug() << "map1 and map2 are not equal.";
}
if (isEqual(map1, map3)) {
qDebug() << "map1 and map3 are equal.";
} else {
qDebug() << "map1 and map3 are not equal.";
}
```
相关推荐
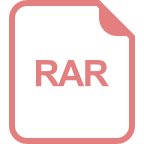
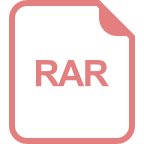
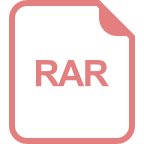














