请写出python线程安全的的单例模式,不要使用饿汉式
时间: 2024-02-16 07:05:22 浏览: 93
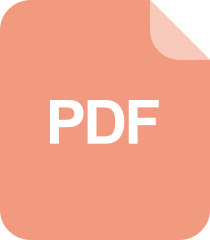
线程安全的单例模式
以下是一个线程安全的Python单例模式实现,使用懒汉式:
```python
import threading
class Singleton:
__instance = None
__lock = threading.Lock()
@classmethod
def get_instance(cls):
if cls.__instance is None:
with cls.__lock:
if cls.__instance is None:
cls.__instance = Singleton()
return cls.__instance
def __init__(self):
if Singleton.__instance is not None:
raise Exception("This class is a singleton!")
else:
Singleton.__instance = self
```
使用方法:
```python
s1 = Singleton.get_instance()
s2 = Singleton.get_instance()
print(s1 is s2) # 输出 True
```
在上面的实现中,使用了一个静态变量`__instance`来保存单例对象,以及一个静态锁`__lock`来保证线程安全。在`get_instance`方法中,首先判断单例对象是否已经存在,如果不存在则加锁创建,保证只有一个线程能够创建成功,最后返回单例对象。在`__init__`方法中,检查单例对象是否已经存在,如果存在则抛出异常,保证单例的唯一性。
阅读全文
相关推荐
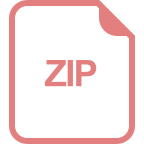
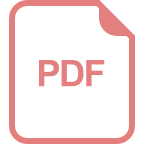
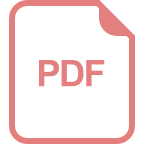
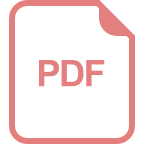
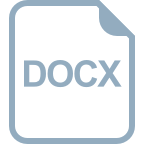
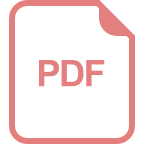
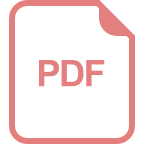
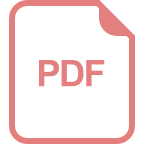
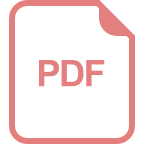
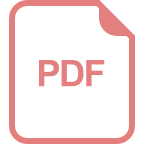
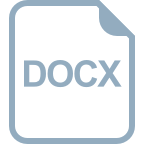
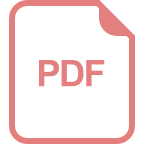
