python实现线程安全的单例模式
时间: 2023-10-08 13:07:55 浏览: 148
在 Python 中,可以使用线程安全的方式实现单例模式。下面是一个示例代码:
```python
import threading
class Singleton:
__instance = None
__lock = threading.Lock()
@staticmethod
def get_instance():
if Singleton.__instance is None:
with Singleton.__lock:
if Singleton.__instance is None:
Singleton.__instance = Singleton()
return Singleton.__instance
def __init__(self):
if Singleton.__instance is not None:
raise Exception("This class is a singleton!")
```
在上面的代码中,使用了一个类变量 `__instance` 来保存单例实例,通过 `get_instance` 方法获取单例对象。通过 `threading.Lock()` 创建一个锁对象 `__lock` 来确保在多线程环境下只有一个线程能够创建单例实例。
在 `get_instance` 方法中,先检查 `__instance` 是否为 None,如果是,则获取锁,再次检查 `__instance` 是否为 None,如果是,则创建单例实例。使用锁可以保证只有一个线程能够进入临界区,确保只有一个实例被创建。
在单例类的构造函数中,可以添加逻辑来防止通过其他方式创建实例,比如抛出异常。
使用示例:
```python
singleton1 = Singleton.get_instance()
singleton2 = Singleton.get_instance()
print(singleton1 is singleton2) # 输出: True
```
以上代码保证了在多线程环境下只会存在一个 Singleton 实例,并且在单例类的构造函数中添加了逻辑防止通过其他方式创建实例。
阅读全文
相关推荐

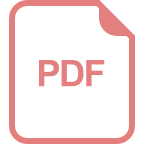
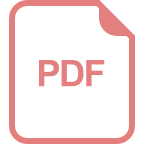
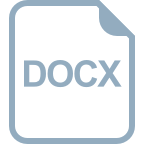
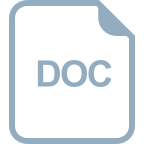
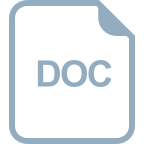
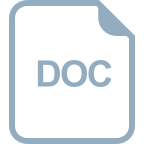
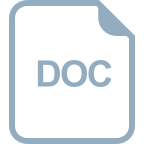
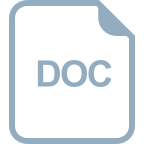
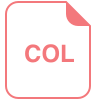




