Java PDF文档上传到服务器涉及代码
时间: 2024-02-02 10:03:45 浏览: 81
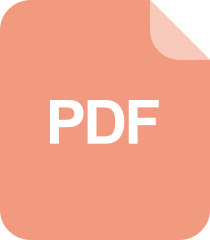
java文件上传客户端和服务器端.pdf
上传Java PDF文档到服务器的代码可以分为两个步骤:文件上传和文件保存。下面是一个简单的示例代码:
文件上传:
```java
@PostMapping("/upload")
public String handleFileUpload(@RequestParam("file") MultipartFile file) {
try {
// 获取文件名
String fileName = StringUtils.cleanPath(file.getOriginalFilename());
// 保存文件到服务器
String uploadDir = "/path/to/upload/directory";
Path uploadPath = Paths.get(uploadDir);
if (!Files.exists(uploadPath)) {
Files.createDirectories(uploadPath);
}
try (InputStream inputStream = file.getInputStream()) {
Path filePath = uploadPath.resolve(fileName);
Files.copy(inputStream, filePath, StandardCopyOption.REPLACE_EXISTING);
}
return "File uploaded successfully!";
} catch (IOException ex) {
return "Error uploading file: " + ex.getMessage();
}
}
```
文件保存:
```java
public void saveFileToDatabase(String fileName, byte[] fileContent) {
try {
// 保存文件到数据库
String jdbcUrl = "jdbc:mysql://localhost:3306/mydb";
String username = "myuser";
String password = "mypassword";
Class.forName("com.mysql.jdbc.Driver");
try (Connection conn = DriverManager.getConnection(jdbcUrl, username, password)) {
String sql = "INSERT INTO files (name, content) VALUES (?, ?)";
try (PreparedStatement stmt = conn.prepareStatement(sql)) {
stmt.setString(1, fileName);
stmt.setBytes(2, fileContent);
stmt.executeUpdate();
}
}
} catch (ClassNotFoundException | SQLException ex) {
ex.printStackTrace();
}
}
```
注意,以上代码仅仅是示例代码,需要根据实际情况进行修改和完善。
阅读全文
相关推荐
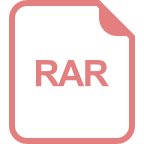
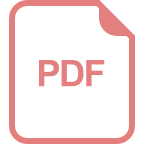















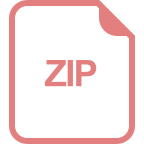