vue 视频九宫格拖动播放
时间: 2023-09-12 18:08:49 浏览: 75
实现九宫格拖动播放视频需要使用 Vue 和一些第三方插件。以下是一个简单的实现步骤:
1. 安装 vue-grid-layout 插件,该插件可以帮助我们实现可拖动的九宫格布局。
```
npm install vue-grid-layout --save
```
2. 在 Vue 组件中引入 vue-grid-layout,并初始化网格布局。具体可以参考 vue-grid-layout 文档。
```html
<template>
<vue-grid-layout :layout="layout" :col-num="3" :row-height="100" :is-draggable="true" :is-resizable="false">
<div v-for="(video, index) in videos" :key="index" :data-grid="{x: video.x, y: video.y, w: video.w, h: video.h}">
<video :src="video.src" controls></video>
</div>
</vue-grid-layout>
</template>
<script>
import VueGridLayout from 'vue-grid-layout'
export default {
components: {
VueGridLayout,
},
data() {
return {
layout: [],
videos: [
{ src: 'video1.mp4', x: 0, y: 0, w: 1, h: 1 },
{ src: 'video2.mp4', x: 1, y: 0, w: 1, h: 1 },
{ src: 'video3.mp4', x: 2, y: 0, w: 1, h: 1 },
{ src: 'video4.mp4', x: 0, y: 1, w: 1, h: 1 },
{ src: 'video5.mp4', x: 1, y: 1, w: 1, h: 1 },
{ src: 'video6.mp4', x: 2, y: 1, w: 1, h: 1 },
{ src: 'video7.mp4', x: 0, y: 2, w: 1, h: 1 },
{ src: 'video8.mp4', x: 1, y: 2, w: 1, h: 1 },
{ src: 'video9.mp4', x: 2, y: 2, w: 1, h: 1 },
],
}
},
created() {
// 初始化九宫格布局
this.layout = this.videos.map(video => {
return { x: video.x, y: video.y, w: video.w, h: video.h, i: String(this.videos.indexOf(video)) }
})
},
}
</script>
```
3. 在 video 标签中添加 controls 属性,可以在视频上添加默认的播放控件。
4. 在组件中处理视频的播放暂停事件。可以使用 videojs 插件,该插件提供了丰富的视频控制 API。
```html
<template>
<vue-grid-layout :layout="layout" :col-num="3" :row-height="100" :is-draggable="true" :is-resizable="false">
<div v-for="(video, index) in videos" :key="index" :data-grid="{x: video.x, y: video.y, w: video.w, h: video.h}">
<video ref="videoPlayer" :src="video.src" controls></video>
</div>
</vue-grid-layout>
</template>
<script>
import VueGridLayout from 'vue-grid-layout'
import videojs from 'video.js'
export default {
components: {
VueGridLayout,
},
data() {
return {
layout: [],
videos: [
{ src: 'video1.mp4', x: 0, y: 0, w: 1, h: 1 },
{ src: 'video2.mp4', x: 1, y: 0, w: 1, h: 1 },
{ src: 'video3.mp4', x: 2, y: 0, w: 1, h: 1 },
{ src: 'video4.mp4', x: 0, y: 1, w: 1, h: 1 },
{ src: 'video5.mp4', x: 1, y: 1, w: 1, h: 1 },
{ src: 'video6.mp4', x: 2, y: 1, w: 1, h: 1 },
{ src: 'video7.mp4', x: 0, y: 2, w: 1, h: 1 },
{ src: 'video8.mp4', x: 1, y: 2, w: 1, h: 1 },
{ src: 'video9.mp4', x: 2, y: 2, w: 1, h: 1 },
],
}
},
created() {
// 初始化九宫格布局
this.layout = this.videos.map(video => {
return { x: video.x, y: video.y, w: video.w, h: video.h, i: String(this.videos.indexOf(video)) }
})
},
mounted() {
// 初始化视频播放器
this.videos.forEach((video, index) => {
const videoPlayer = videojs(this.$refs[`videoPlayer${index}`], {}, function onPlayerReady() {
videoPlayer.on('play', () => {
console.log('Video is playing')
})
videoPlayer.on('pause', () => {
console.log('Video is paused')
})
})
})
},
}
</script>
```
注意:上述代码中的 `videojs` 插件需要单独安装。
```
npm install video.js --save
```
以上就是使用 Vue 和第三方插件实现九宫格拖动播放视频的基本步骤。当然,您可以根据自己的需求进行扩展和优化。
相关推荐












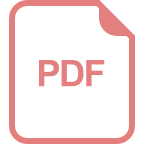
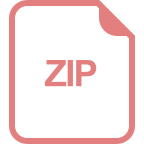