不是这个意思,是给出包含fopen函数的读写文本文件和二进制文件的示例代码
时间: 2024-03-23 15:41:18 浏览: 65
非常抱歉,我理解有误。下面是包含 `fopen` 函数的读写文本文件和二进制文件的示例代码:
1. 读写文本文件:
```c++
#include <stdio.h>
int main() {
FILE* fp;
char buf[512];
// 以只读方式打开文件
fp = fopen("example.txt", "r");
if (!fp) {
printf("Failed to open file!\n");
return 0;
}
// 读取文件内容
while (fgets(buf, sizeof(buf), fp)) {
printf("%s", buf);
}
// 关闭文件
fclose(fp);
// 以只写方式打开文件
fp = fopen("example.txt", "a");
if (!fp) {
printf("Failed to open file!\n");
return 0;
}
// 写入文件内容
fprintf(fp, "This is a new line.\n");
// 关闭文件
fclose(fp);
return 0;
}
```
在以上示例代码中,`fopen` 函数用于打开文件,`fgets` 函数用于读取文件内容,`fprintf` 函数用于写入文件内容,`fclose` 函数用于关闭文件。
2. 读写二进制文件:
```c++
#include <stdio.h>
struct student {
int id;
char name[20];
double score;
};
int main() {
FILE* fp;
student s;
// 以二进制读方式打开文件
fp = fopen("example.bin", "rb");
if (!fp) {
printf("Failed to open file!\n");
return 0;
}
// 读取文件内容
while (fread(&s, sizeof(s), 1, fp)) {
printf("%d %s %lf\n", s.id, s.name, s.score);
}
// 关闭文件
fclose(fp);
// 以二进制写方式打开文件
fp = fopen("example.bin", "ab");
if (!fp) {
printf("Failed to open file!\n");
return 0;
}
// 写入文件内容
student s1 = {1, "Tom", 90.5};
student s2 = {2, "Jerry", 85.5};
fwrite(&s1, sizeof(s1), 1, fp);
fwrite(&s2, sizeof(s2), 1, fp);
// 关闭文件
fclose(fp);
return 0;
}
```
在以上示例代码中,`fopen` 函数用于打开文件,`fread` 函数用于读取文件内容,`fwrite` 函数用于写入文件内容,`fclose` 函数用于关闭文件。注意,二进制文件需要以二进制方式打开,因此在文件打开模式中需要加上 "b" 标识。
阅读全文
相关推荐
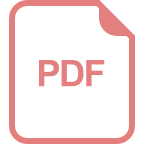

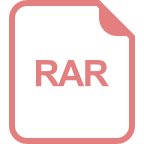
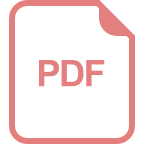
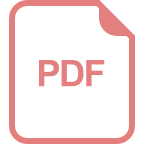
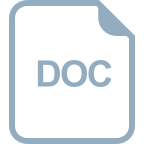
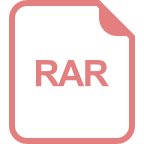
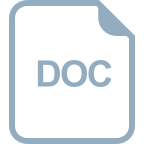
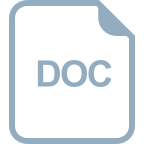
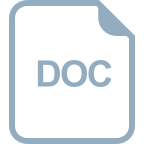
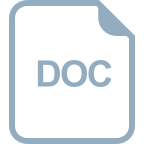
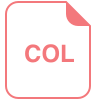




