帮我用Html写一个时钟的页面
时间: 2023-09-13 12:04:49 浏览: 69
### 回答1:
好的,以下是一个用HTML写的时钟页面的示例代码:
```
<!DOCTYPE html>
<html>
<head>
<title>时钟</title>
<style>
#clock {
font-size: 100px;
font-weight: bold;
text-align: center;
margin-top: 100px;
}
</style>
</head>
<body onload="startTime()">
<div id="clock"></div>
<script>
function startTime() {
var today = new Date();
var h = today.getHours();
var m = today.getMinutes();
var s = today.getSeconds();
m = checkTime(m);
s = checkTime(s);
document.getElementById('clock').innerHTML = h + ":" + m + ":" + s;
setTimeout(startTime, 1000);
}
function checkTime(i) {
if (i < 10) {i = "0" + i};
return i;
}
</script>
</body>
</html>
```
这段代码会在页面上创建一个时钟,并且实时更新显示当前的时间。其中,通过`<div>`元素来创建时钟的界面,使用了CSS样式来设置字体大小、字体加粗、文本对齐以及上边距。通过JavaScript中的`Date()`函数获取当前时间,再通过`innerHTML`属性将时、分、秒信息显示到页面上。最后,使用`setTimeout()`函数实现每隔一秒钟更新一次时间。
你可以将这段代码复制到文本编辑器中,并将其保存为`.html`文件,然后在浏览器中打开该文件以查看效果。
### 回答2:
当然可以帮您编写一个用HTML制作的时钟页面。
首先,在HTML中创建一个div元素来放置时钟的数字显示部分。代码如下所示:
```html
<div id="clock"></div>
```
接下来,您可以使用JavaScript来创建一个函数,用来实时更新时钟的时间。代码如下:
```html
<script>
// 获取当前时间
function updateClock() {
var now = new Date();
var hours = now.getHours();
var minutes = now.getMinutes();
var seconds = now.getSeconds();
// 将时间格式化为两位数
hours = formatTime(hours);
minutes = formatTime(minutes);
seconds = formatTime(seconds);
// 将时间显示在页面上
var clock = document.getElementById("clock");
clock.innerHTML = hours + ":" + minutes + ":" + seconds;
// 每秒更新一次时间
setTimeout(updateClock, 1000);
}
// 格式化时间
function formatTime(time) {
if (time < 10) {
return "0" + time;
} else {
return time;
}
}
// 在页面加载完成后开始更新时间
window.onload = function() {
updateClock();
};
</script>
```
最后,您只需在页面底部插入上述代码,并将此HTML文件保存为一个.html文件,即可在浏览器中打开查看时钟页面。
希望以上回答能对您有所帮助。如果您有其他问题,欢迎随时提问。
### 回答3:
当然可以!下面是一个简单的HTML页面,展示了一个基本的时钟功能:
```html
<!DOCTYPE html>
<html>
<head>
<title>时钟页面</title>
<style>
/* 样式设置 */
body {
text-align: center;
margin-top: 100px;
font-size: 48px;
}
</style>
</head>
<body>
<div id="clock">00:00:00</div> <!-- 显示时间的div元素 -->
<script>
// 更新时间的函数
function updateTime() {
var currentTime = new Date(); // 获取当前时间
var hours = currentTime.getHours();
var minutes = currentTime.getMinutes();
var seconds = currentTime.getSeconds();
// 数字小于 10 时,在数字前面加上一个 '0'
hours = (hours < 10 ? "0" : "") + hours;
minutes = (minutes < 10 ? "0" : "") + minutes;
seconds = (seconds < 10 ? "0" : "") + seconds;
// 将时间显示在页面上
document.getElementById('clock').innerHTML = hours + ":" + minutes + ":" + seconds;
}
// 每秒更新一次时间
setInterval(updateTime, 1000);
</script>
</body>
</html>
```
这个页面会显示一个居中的大字时钟,通过调用 JavaScript 动态更新时间。简单通过 HTML、CSS 和 JavaScript 来实现。希望对你有帮助!
相关推荐
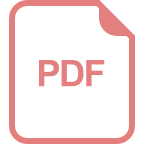













