APP\ws2812\ws2812.c(63): warning: #223-D: function "GPIO_SetBits" declared implicitly
时间: 2024-01-20 12:04:15 浏览: 33
这个警告信息的意思是在第63行代码中的函数“GPIO_SetBits”在使用前没有进行显式声明。这通常是因为您没有包含该函数所在的头文件,或者没有在程序中正确声明该函数。
解决此问题的方法是确保在使用该函数之前,已经包含了该函数所在的头文件,并且已经正确声明了该函数。例如,如果您使用的是STM32CubeMX生成的代码,则可以在main函数的开头添加以下代码:
```c
#include "stm32f4xx_hal_gpio.h"
```
这将包含GPIO库的头文件,其中包含了GPIO_SetBits函数的声明。如果您使用的是其他库或自己编写的代码,请确保按照库的文档进行正确的包含和声明。
相关问题
..\user\ADX922.c(117): warning: #223-D: function "GPIO_ResetBits" declared implicitly
这个警告是因为在你的代码中使用了 `GPIO_ResetBits` 函数,但是该函数在当前文件中没有被声明。为了消除这个警告,你需要在代码的开头添加一个函数原型声明,或者在代码中包含该函数的头文件。例如:
```c
#include "stm32f10x_gpio.h" // 包含 GPIO 头文件
// 声明函数原型
void GPIO_ResetBits(GPIO_TypeDef* GPIOx, uint16_t GPIO_Pin);
int main(void)
{
// ...
GPIO_ResetBits(GPIOA, GPIO_Pin_0);
// ...
}
// 实现函数
void GPIO_ResetBits(GPIO_TypeDef* GPIOx, uint16_t GPIO_Pin)
{
// ...
}
```
这样,编译器就知道 `GPIO_ResetBits` 函数的声明,就不会再给出警告了。
User\main.c(23): warning: #223-D: function "motor_gpio" declared implicitly
这个警告意味着在 `main.c` 文件中,函数 `motor_gpio` 没有被正确地声明。这可能是因为你在调用 `motor_gpio` 函数之前没有在代码中声明该函数。为了解决这个警告,你需要在 `main.c` 文件中添加一个函数声明,以便编译器知道如何正确处理 `motor_gpio` 函数。例如,如果 `motor_gpio` 函数的原型如下:
```c
void motor_gpio(void);
```
那么你需要在 `main.c` 文件中添加以下函数声明:
```c
void motor_gpio(void);
```
将此函数声明放在 `main` 函数之前,这样编译器就知道 `motor_gpio` 函数的签名并且可以正确编译你的代码。
相关推荐
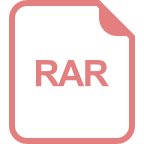
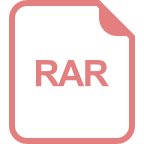
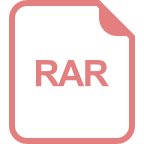













