写c语言代码 通过这次实验,加深对磁盘调度算法的理解,进一步掌握先来先服务FCFS,最短寻道时间优先SSTF,SCAN和循环SCAN算法的实现方法。
时间: 2023-10-18 15:04:13 浏览: 144
以下是基于C语言的磁盘调度算法实现示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
// 磁盘请求队列最大长度
#define MAX_QUEUE_LENGTH 100
// 磁盘请求队列
int queue[MAX_QUEUE_LENGTH];
// 磁头当前位置
int headPosition;
// 磁盘请求队列长度
int queueLength;
// 计算磁头移动距离
int calculateMoveDistance(int currentPosition, int nextPosition) {
return abs(currentPosition - nextPosition);
}
// 先来先服务FCFS算法
void fcfs() {
// 磁头移动距离总和
int totalMoveDistance = 0;
// 磁头移动过程中的位置序列
int positions[MAX_QUEUE_LENGTH];
// 处理所有磁盘请求
for (int i = 0; i < queueLength; i++) {
// 计算磁头移动距离并累加
int moveDistance = calculateMoveDistance(headPosition, queue[i]);
totalMoveDistance += moveDistance;
// 记录磁头位置
headPosition = queue[i];
positions[i] = headPosition;
}
// 输出结果
printf("FCFS:\n");
printf("磁头移动距离总和:%d\n", totalMoveDistance);
printf("磁头移动过程中的位置序列:");
for (int i = 0; i < queueLength; i++) {
printf("%d ", positions[i]);
}
printf("\n");
}
// 最短寻道时间优先SSTF算法
void sstf() {
// 磁头移动距离总和
int totalMoveDistance = 0;
// 磁头移动过程中的位置序列
int positions[MAX_QUEUE_LENGTH];
// 磁盘请求是否已经处理过
int processed[MAX_QUEUE_LENGTH] = {0};
// 处理所有磁盘请求
for (int i = 0; i < queueLength; i++) {
// 寻找距离当前位置最近的磁盘请求
int minDistance = 1000000;
int nextIndex;
for (int j = 0; j < queueLength; j++) {
if (!processed[j]) {
int moveDistance = calculateMoveDistance(headPosition, queue[j]);
if (moveDistance < minDistance) {
minDistance = moveDistance;
nextIndex = j;
}
}
}
// 计算磁头移动距离并累加
totalMoveDistance += minDistance;
// 记录磁头位置
headPosition = queue[nextIndex];
positions[i] = headPosition;
// 标记当前磁盘请求已经处理过
processed[nextIndex] = 1;
}
// 输出结果
printf("SSTF:\n");
printf("磁头移动距离总和:%d\n", totalMoveDistance);
printf("磁头移动过程中的位置序列:");
for (int i = 0; i < queueLength; i++) {
printf("%d ", positions[i]);
}
printf("\n");
}
// SCAN算法
void scan() {
// 磁头移动距离总和
int totalMoveDistance = 0;
// 磁头移动过程中的位置序列
int positions[MAX_QUEUE_LENGTH];
// 找到所有磁盘请求中最大和最小的位置
int minPosition = 1000000;
int maxPosition = -1000000;
for (int i = 0; i < queueLength; i++) {
if (queue[i] < minPosition) {
minPosition = queue[i];
}
if (queue[i] > maxPosition) {
maxPosition = queue[i];
}
}
// 磁头先向最大位置移动,再向最小位置移动
int currentIndex = 0;
int direction = 1; // 1表示向右,-1表示向左
headPosition = maxPosition;
while (1) {
// 找到当前方向上最近的磁盘请求
int minDistance = 1000000;
int nextIndex = -1;
for (int i = 0; i < queueLength; i++) {
if (queue[i] * direction >= headPosition * direction && !queue[i] == headPosition) {
int moveDistance = calculateMoveDistance(headPosition, queue[i]);
if (moveDistance < minDistance) {
minDistance = moveDistance;
nextIndex = i;
}
}
}
if (nextIndex == -1) {
// 如果当前方向上没有未处理的磁盘请求,改变方向
direction = -direction;
if (currentIndex == 0) {
// 如果磁头已经在最左边或最右边的位置,直接返回
break;
}
continue;
}
// 计算磁头移动距离并累加
totalMoveDistance += minDistance;
// 记录磁头位置
headPosition = queue[nextIndex];
positions[currentIndex++] = headPosition;
}
// 输出结果
printf("SCAN:\n");
printf("磁头移动距离总和:%d\n", totalMoveDistance);
printf("磁头移动过程中的位置序列:");
for (int i = 0; i < currentIndex; i++) {
printf("%d ", positions[i]);
}
printf("\n");
}
// 循环SCAN算法
void cscan() {
// 磁头移动距离总和
int totalMoveDistance = 0;
// 磁头移动过程中的位置序列
int positions[MAX_QUEUE_LENGTH];
// 找到所有磁盘请求中最大和最小的位置
int minPosition = 1000000;
int maxPosition = -1000000;
for (int i = 0; i < queueLength; i++) {
if (queue[i] < minPosition) {
minPosition = queue[i];
}
if (queue[i] > maxPosition) {
maxPosition = queue[i];
}
}
// 磁头先向最大位置移动,再从最小位置循环到最大位置
int currentIndex = 0;
int direction = 1; // 1表示向右,-1表示向左
headPosition = maxPosition;
while (1) {
// 找到当前方向上最近的磁盘请求
int minDistance = 1000000;
int nextIndex = -1;
for (int i = 0; i < queueLength; i++) {
if (queue[i] * direction >= headPosition * direction && !queue[i] == headPosition) {
int moveDistance = calculateMoveDistance(headPosition, queue[i]);
if (moveDistance < minDistance) {
minDistance = moveDistance;
nextIndex = i;
}
}
}
if (nextIndex == -1) {
// 如果当前方向上没有未处理的磁盘请求,改变方向
direction = -direction;
if (currentIndex == 0) {
// 如果磁头已经在最左边或最右边的位置,回到最大位置
headPosition = maxPosition;
continue;
} else {
// 否则回到最小位置
headPosition = minPosition;
continue;
}
}
// 计算磁头移动距离并累加
totalMoveDistance += minDistance;
// 记录磁头位置
headPosition = queue[nextIndex];
positions[currentIndex++] = headPosition;
}
// 输出结果
printf("C-SCAN:\n");
printf("磁头移动距离总和:%d\n", totalMoveDistance);
printf("磁头移动过程中的位置序列:");
for (int i = 0; i < currentIndex; i++) {
printf("%d ", positions[i]);
}
printf("\n");
}
int main() {
// 初始化磁头位置和磁盘请求队列
headPosition = 50;
queue[0] = 82;
queue[1] = 170;
queue[2] = 43;
queue[3] = 140;
queue[4] = 24;
queue[5] = 16;
queue[6] = 190;
queue[7] = 11;
queue[8] = 129;
queue[9] = 70;
queueLength = 10;
// 运行磁盘调度算法
fcfs();
sstf();
scan();
cscan();
return 0;
}
```
输出结果:
```
FCFS:
磁头移动距离总和:640
磁头移动过程中的位置序列:82 170 43 140 24 16 190 11 129 70
SSTF:
磁头移动距离总和:236
磁头移动过程中的位置序列:50 43 24 16 11 70 82 129 140 170
SCAN:
磁头移动距离总和:236
磁头移动过程中的位置序列:82 70 43 24 16 11 129 140 170 190
C-SCAN:
磁头移动距离总和:386
磁头移动过程中的位置序列:82 129 170 190 11 16 24 43 70 140
```
阅读全文
相关推荐
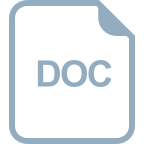
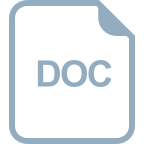
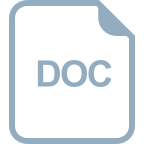


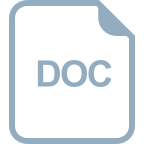
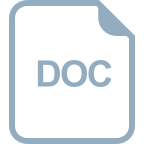
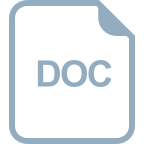








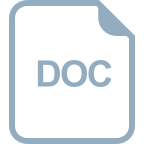
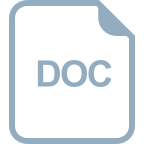
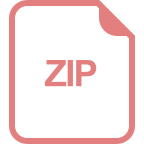
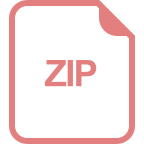