c++定义一个抽象类Shape,有2个纯虚函数计算体积和表面积:calVolume()和calArea(),返回值为double型。由Shape类派生出3种几何图形:正方体类,球体类和圆柱体类,各自新增的数据成员均为double型。 对于calVolume()和calArea(),每一个定义两个普通的重载函数:返回值均为void,形参分别是抽象类的对象指针、抽象类的对象引用,在函数中通过指针或引用调用虚函数calArea()或者calVolume(),输出结果。 在main函数中,进行测试,必须包含重载函
时间: 2024-02-15 18:05:58 浏览: 121
数的调用。代码如下:
```c++
#include <iostream>
using namespace std;
class Shape {
public:
virtual double calVolume() = 0;
virtual double calArea() = 0;
virtual ~Shape() {}
};
class Cube : public Shape {
public:
Cube(double l) : length(l) {}
double calVolume() { return length * length * length; }
double calArea() { return 6 * length * length; }
private:
double length;
};
class Sphere : public Shape {
public:
Sphere(double r) : radius(r) {}
double calVolume() { return 4.0 / 3.0 * 3.1415926 * radius * radius * radius; }
double calArea() { return 4 * 3.1415926 * radius * radius; }
private:
double radius;
};
class Cylinder : public Shape {
public:
Cylinder(double r, double h) : radius(r), height(h) {}
double calVolume() { return 3.1415926 * radius * radius * height; }
double calArea() { return 2 * 3.1415926 * radius * height + 2 * 3.1415926 * radius * radius; }
private:
double radius;
double height;
};
void calVolume(Shape* s) {
cout << "The volume of the shape is " << s->calVolume() << endl;
}
void calArea(Shape& s) {
cout << "The area of the shape is " << s.calArea() << endl;
}
int main() {
Cube c(2);
Sphere sp(3);
Cylinder cy(2, 5);
calVolume(&c);
calArea(c);
calVolume(&sp);
calArea(sp);
calVolume(&cy);
calArea(cy);
return 0;
}
```
阅读全文
相关推荐
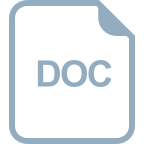
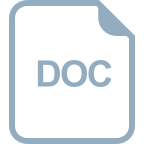
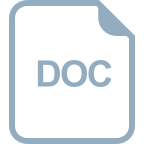














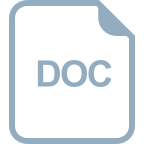
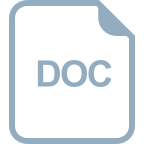