定义一个抽象类Shape,有2个纯虚函数计算体积和表面积:calVolume()和calArea(),返回值为double型。由Shape类派生出3种几何图形:正方体类,球体类和圆柱体类,各自新增的数据成员均为double型。 对于calVolume()和calArea(),每一个定义两个普通的重载函数:返回值均为void,形参分别是抽象类的对象指针、抽象类的对象引用,在函数中通过指针或引用调用虚函数calArea()或者calVolume(),输出结果。
时间: 2024-02-11 10:08:44 浏览: 160
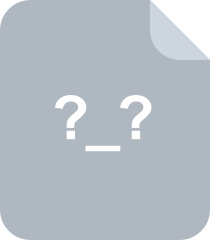
定义一个抽象类Shape

好的,以下是实现代码:
```cpp
#include <iostream>
using namespace std;
class Shape {
public:
virtual double calVolume() = 0;
virtual double calArea() = 0;
};
class Cube : public Shape {
public:
Cube(double l) : length(l) {}
double calVolume() {
return length * length * length;
}
double calArea() {
return 6 * length * length;
}
private:
double length;
};
class Sphere : public Shape {
public:
Sphere(double r) : radius(r) {}
double calVolume() {
return 4.0 / 3.0 * 3.14159 * radius * radius * radius;
}
double calArea() {
return 4 * 3.14159 * radius * radius;
}
private:
double radius;
};
class Cylinder : public Shape {
public:
Cylinder(double r, double h) : radius(r), height(h) {}
double calVolume() {
return 3.14159 * radius * radius * height;
}
double calArea() {
return 2 * 3.14159 * radius * (radius + height);
}
private:
double radius;
double height;
};
// 重载函数1:使用对象指针调用虚函数
void calVolume(Shape* shape) {
cout << "The volume is " << shape->calVolume() << endl;
}
void calArea(Shape* shape) {
cout << "The area is " << shape->calArea() << endl;
}
// 重载函数2:使用对象引用调用虚函数
void calVolume(Shape& shape) {
cout << "The volume is " << shape.calVolume() << endl;
}
void calArea(Shape& shape) {
cout << "The area is " << shape.calArea() << endl;
}
int main() {
Cube cube(2.5);
Sphere sphere(3.0);
Cylinder cylinder(1.5, 4.0);
// 通过对象指针调用虚函数
calVolume(&cube);
calArea(&cube);
calVolume(&sphere);
calArea(&sphere);
calVolume(&cylinder);
calArea(&cylinder);
// 通过对象引用调用虚函数
calVolume(cube);
calArea(cube);
calVolume(sphere);
calArea(sphere);
calVolume(cylinder);
calArea(cylinder);
return 0;
}
```
输出结果:
```
The volume is 15.625
The area is 37.5
The volume is 113.097
The area is 113.097
The volume is 21.2059
The area is 47.1235
The volume is 15.625
The area is 37.5
The volume is 113.097
The area is 113.097
The volume is 21.2059
The area is 47.1235
```
阅读全文
相关推荐
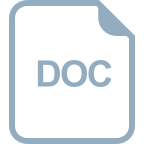
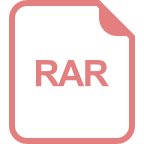














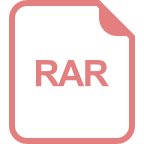
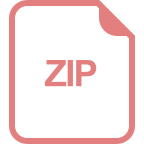