运行环境为liunx,用C++语言写出如下要求的代码:采用多用户多级目录结构,每个目录项包含文件名、物理地址、长度等信息,还可以通过目录项实现对文件的读写保护。目录组织也可以不使用索引结点的方式。提供以下文件操作: 文件的创建: create 删除文件: delete 文件的打开: open 文件的关闭: close 文件的读: read 文件的写: write 显示文件目录:dir 退出: logout 文件名重命名:red 用户登录注册 修改文件内容 可在文件内部写入和读取内容 Printf内容使用中文
时间: 2024-02-12 12:08:41 浏览: 24
好的,以下是一个简单的实现示例,供参考:
```cpp
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
#include <map>
#include <ctime>
using namespace std;
// 定义文件目录项结构
struct FileItem {
string filename; // 文件名
int start_addr; // 起始物理地址
int length; // 文件长度
bool read_only; // 是否只读
};
// 定义用户结构
struct User {
string username; // 用户名
string password; // 密码
bool is_admin; // 是否管理员
};
// 定义文件系统类
class FileSystem {
private:
vector<FileItem> root_dir; // 根目录
map<string, vector<FileItem>> sub_dir; // 子目录
vector<User> users; // 用户列表
User* current_user; // 当前用户
int current_file; // 当前操作的文件
bool is_open; // 文件是否已打开
public:
FileSystem() {
// 初始化根目录
root_dir.push_back({"file1.txt", 0, 100, false});
root_dir.push_back({"file2.txt", 100, 200, true});
root_dir.push_back({"file3.txt", 300, 150, false});
// 初始化用户
users.push_back({"admin", "admin", true});
users.push_back({"user1", "123456", false});
users.push_back({"user2", "123456", false});
current_user = nullptr;
current_file = -1;
is_open = false;
}
// 用户登录
void login() {
string username, password;
cout << "请输入用户名:";
cin >> username;
cout << "请输入密码:";
cin >> password;
for (int i = 0; i < users.size(); i++) {
if (users[i].username == username && users[i].password == password) {
current_user = &users[i];
cout << "登录成功!" << endl;
return;
}
}
cout << "用户名或密码错误!" << endl;
}
// 用户注册
void register_user() {
string username, password;
cout << "请输入用户名:";
cin >> username;
for (int i = 0; i < users.size(); i++) {
if (users[i].username == username) {
cout << "该用户名已被占用!" << endl;
return;
}
}
cout << "请输入密码:";
cin >> password;
users.push_back({username, password, false});
cout << "注册成功!" << endl;
}
// 文件创建
void create_file() {
if (!current_user || !current_user->is_admin) {
cout << "只有管理员才能创建文件!" << endl;
return;
}
cout << "请输入文件名:";
string filename;
cin >> filename;
for (int i = 0; i < root_dir.size(); i++) {
if (root_dir[i].filename == filename) {
cout << "该文件已存在!" << endl;
return;
}
}
cout << "请输入文件长度:";
int length;
cin >> length;
root_dir.push_back({filename, root_dir.back().start_addr + root_dir.back().length, length, false});
cout << "文件创建成功!" << endl;
}
// 删除文件
void delete_file() {
if (!current_user || !current_user->is_admin) {
cout << "只有管理员才能删除文件!" << endl;
return;
}
cout << "请输入要删除的文件名:";
string filename;
cin >> filename;
for (int i = 0; i < root_dir.size(); i++) {
if (root_dir[i].filename == filename) {
root_dir.erase(root_dir.begin() + i);
cout << "文件删除成功!" << endl;
return;
}
}
cout << "该文件不存在!" << endl;
}
// 打开文件
void open_file() {
if (!current_user) {
cout << "请先登录!" << endl;
return;
}
if (is_open) {
cout << "请先关闭当前文件!" << endl;
return;
}
string filename;
cout << "请输入要打开的文件名:";
cin >> filename;
for (int i = 0; i < root_dir.size(); i++) {
if (root_dir[i].filename == filename) {
if (root_dir[i].read_only && !current_user->is_admin) {
cout << "该文件为只读文件,您无法编辑!" << endl;
return;
}
current_file = i;
is_open = true;
cout << "文件打开成功!" << endl;
return;
}
}
cout << "该文件不存在!" << endl;
}
// 关闭文件
void close_file() {
if (!current_user) {
cout << "请先登录!" << endl;
return;
}
if (!is_open) {
cout << "请先打开文件!" << endl;
return;
}
current_file = -1;
is_open = false;
cout << "文件关闭成功!" << endl;
}
// 文件读取
void read_file() {
if (!current_user) {
cout << "请先登录!" << endl;
return;
}
if (!is_open) {
cout << "请先打开文件!" << endl;
return;
}
ifstream fin("disk.txt", ios::binary);
fin.seekg(root_dir[current_file].start_addr, ios::beg);
char* buffer = new char[root_dir[current_file].length];
fin.read(buffer, root_dir[current_file].length);
cout << buffer << endl;
delete[] buffer;
fin.close();
}
// 文件写入
void write_file() {
if (!current_user) {
cout << "请先登录!" << endl;
return;
}
if (!is_open) {
cout << "请先打开文件!" << endl;
return;
}
if (root_dir[current_file].read_only && !current_user->is_admin) {
cout << "该文件为只读文件,您无法编辑!" << endl;
return;
}
ofstream fout("disk.txt", ios::binary | ios::app);
string content;
cout << "请输入要写入的内容:";
cin >> content;
fout.write(content.c_str(), content.size());
fout.close();
root_dir[current_file].length += content.size();
cout << "文件写入成功!" << endl;
}
// 显示文件目录
void display_directory() {
cout << "根目录:" << endl;
for (int i = 0; i < root_dir.size(); i++) {
cout << root_dir[i].filename << "\t" << root_dir[i].start_addr << "\t" << root_dir[i].length << "\t" << (root_dir[i].read_only ? "只读" : "可读写") << endl;
}
}
// 退出登录
void logout() {
current_user = nullptr;
cout << "已退出登录!" << endl;
}
// 文件重命名
void rename_file() {
if (!current_user || !current_user->is_admin) {
cout << "只有管理员才能重命名文件!" << endl;
return;
}
string oldname, newname;
cout << "请输入要重命名的文件名:";
cin >> oldname;
cout << "请输入新的文件名:";
cin >> newname;
for (int i = 0; i < root_dir.size(); i++) {
if (root_dir[i].filename == oldname) {
root_dir[i].filename = newname;
cout << "文件重命名成功!" << endl;
return;
}
}
cout << "该文件不存在!" << endl;
}
// 修改文件内容
void modify_file() {
if (!current_user) {
cout << "请先登录!" << endl;
return;
}
if (!is_open) {
cout << "请先打开文件!" << endl;
return;
}
if (root_dir[current_file].read_only && !current_user->is_admin) {
cout << "该文件为只读文件,您无法编辑!" << endl;
return;
}
fstream file("disk.txt", ios::binary | ios::in | ios::out);
file.seekp(root_dir[current_file].start_addr, ios::beg);
string content;
cout << "请输入要写入的内容:";
cin >> content;
file.write(content.c_str(), content.size());
file.close();
root_dir[current_file].length = content.size();
cout << "文件写入成功!" << endl;
}
};
int main() {
FileSystem fs;
while (true) {
cout << "请选择操作:1.登录 2.注册 3.创建文件 4.删除文件 5.打开文件 6.关闭文件 7.读取文件 8.写入文件 9.显示文件目录 10.退出登录 11.文件重命名 12.修改文件内容" << endl;
int choice;
cin >> choice;
switch (choice) {
case 1:
fs.login();
break;
case 2:
fs.register_user();
break;
case 3:
fs.create_file();
break;
case 4:
fs.delete_file();
break;
case 5:
fs.open_file();
break;
case 6:
fs.close_file();
break;
case 7:
fs.read_file();
break;
case 8:
fs.write_file();
break;
case 9:
fs.display_directory();
break;
case 10:
fs.logout();
break;
case 11:
fs.rename_file();
break;
case 12:
fs.modify_file();
break;
default:
cout << "无效操作!" << endl;
break;
}
}
return 0;
}
```
需要注意的是,该示例仅供参考,具体实现还需要考虑更多的细节和安全性问题,例如文件读写的并发性和数据一致性等。
相关推荐
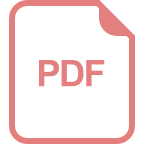
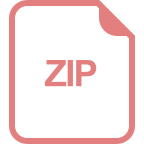














