定义一个简单形体(OpenGL提供的基础形体,如立方体、球体等),并生成正交投影及透视投影视图
时间: 2023-11-22 10:55:48 浏览: 52
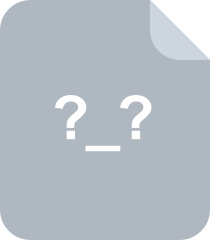
基于OpenGL的计算机图形学实验七定义一个简单形体(OpenGL提供的基础形体,立方体、球体等),并生成正交投影及透视投影视图
好的,以下是一个简单的例子,展示如何在OpenGL中生成立方体,并在正交投影和透视投影下查看:
首先,我们需要包含一些OpenGL头文件和GLUT库:
```c
#include <GL/gl.h>
#include <GL/glu.h>
#include <GL/glut.h>
```
接下来,我们定义一个立方体的顶点坐标数组:
```c
GLfloat vertices[][3] = {{-1.0,-1.0,-1.0},{1.0,-1.0,-1.0},
{1.0,1.0,-1.0},{-1.0,1.0,-1.0},
{-1.0,-1.0,1.0},{1.0,-1.0,1.0},
{1.0,1.0,1.0},{-1.0,1.0,1.0}};
```
然后,我们定义一个绘制立方体的函数:
```c
void drawCube() {
glBegin(GL_QUADS);
// front face
glColor3f(1.0, 0.0, 0.0); // red
glVertex3fv(vertices[0]);
glVertex3fv(vertices[1]);
glVertex3fv(vertices[2]);
glVertex3fv(vertices[3]);
// back face
glColor3f(0.0, 1.0, 0.0); // green
glVertex3fv(vertices[4]);
glVertex3fv(vertices[5]);
glVertex3fv(vertices[6]);
glVertex3fv(vertices[7]);
// top face
glColor3f(0.0, 0.0, 1.0); // blue
glVertex3fv(vertices[3]);
glVertex3fv(vertices[2]);
glVertex3fv(vertices[6]);
glVertex3fv(vertices[7]);
// bottom face
glColor3f(1.0, 1.0, 0.0); // yellow
glVertex3fv(vertices[0]);
glVertex3fv(vertices[1]);
glVertex3fv(vertices[5]);
glVertex3fv(vertices[4]);
// right face
glColor3f(1.0, 0.0, 1.0); // magenta
glVertex3fv(vertices[1]);
glVertex3fv(vertices[5]);
glVertex3fv(vertices[6]);
glVertex3fv(vertices[2]);
// left face
glColor3f(0.0, 1.0, 1.0); // cyan
glVertex3fv(vertices[0]);
glVertex3fv(vertices[4]);
glVertex3fv(vertices[7]);
glVertex3fv(vertices[3]);
glEnd();
}
```
现在,我们可以绘制立方体了。在主函数中,我们首先设置OpenGL的一些参数,然后绘制立方体:
```c
void display() {
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
// 设置正交投影
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
glOrtho(-2.0, 2.0, -2.0, 2.0, -2.0, 2.0);
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
glRotatef(45.0, 1.0, 1.0, 1.0); // 绕 x=y=z 轴旋转 45 度
drawCube();
// 设置透视投影
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
gluPerspective(60.0, 1.0, 1.0, 10.0);
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
gluLookAt(0.0, 0.0, 5.0, 0.0, 0.0, 0.0, 0.0, 1.0, 0.0); // 视点在 (0,0,5), 视线指向原点,向上方向为 y 轴正方向
glTranslatef(0.0, 0.0, -3.0); // 将立方体向后移动 3 个单位
drawCube();
glutSwapBuffers();
}
```
最后,在主函数中启动OpenGL和GLUT:
```c
int main(int argc, char** argv) {
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_DOUBLE | GLUT_RGB | GLUT_DEPTH);
glutInitWindowSize(400, 400);
glutCreateWindow("Cube");
glEnable(GL_DEPTH_TEST);
glutDisplayFunc(display);
glutMainLoop();
return 0;
}
```
现在,你可以编译并运行代码,看看结果如何了。
阅读全文
相关推荐




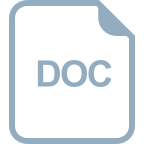
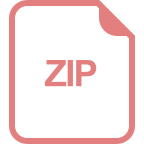
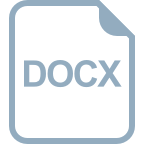
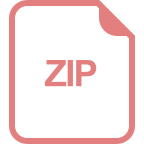
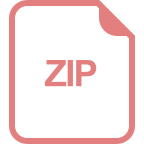