定义一个简单形体(OpenGL提供的基础形体,如立方体、球体等),并生成正交投影及透视投影视图,使用OpenGL等图形库实现; 可交互,可调整观察姿态及位置(调整相机位置、姿态等); 无需考虑斜平行投影;
时间: 2023-07-31 07:08:54 浏览: 70
这里提供一个使用OpenGL库实现的正交投影和透视投影的立方体示例代码,可以通过键盘控制相机位置和姿态:
```c++
#include <GL/glut.h>
#include <stdio.h>
float cameraPos[] = { 0.0, 0.0, 5.0 }; // 相机位置
float cameraFront[] = { 0.0, 0.0, -1.0 }; // 相机前方向
float cameraUp[] = { 0.0, 1.0, 0.0 }; // 相机上方向
void drawCube() // 绘制立方体
{
glBegin(GL_QUADS);
// 前面
glColor3f(1.0, 0.0, 0.0);
glVertex3f(-1.0, -1.0, 1.0);
glVertex3f(1.0, -1.0, 1.0);
glVertex3f(1.0, 1.0, 1.0);
glVertex3f(-1.0, 1.0, 1.0);
// 后面
glColor3f(0.0, 1.0, 0.0);
glVertex3f(-1.0, -1.0, -1.0);
glVertex3f(-1.0, 1.0, -1.0);
glVertex3f(1.0, 1.0, -1.0);
glVertex3f(1.0, -1.0, -1.0);
// 左侧
glColor3f(0.0, 0.0, 1.0);
glVertex3f(-1.0, -1.0, -1.0);
glVertex3f(-1.0, -1.0, 1.0);
glVertex3f(-1.0, 1.0, 1.0);
glVertex3f(-1.0, 1.0, -1.0);
// 右侧
glColor3f(1.0, 1.0, 0.0);
glVertex3f(1.0, -1.0, -1.0);
glVertex3f(1.0, 1.0, -1.0);
glVertex3f(1.0, 1.0, 1.0);
glVertex3f(1.0, -1.0, 1.0);
// 顶部
glColor3f(1.0, 0.0, 1.0);
glVertex3f(-1.0, 1.0, -1.0);
glVertex3f(-1.0, 1.0, 1.0);
glVertex3f(1.0, 1.0, 1.0);
glVertex3f(1.0, 1.0, -1.0);
// 底部
glColor3f(0.0, 1.0, 1.0);
glVertex3f(-1.0, -1.0, -1.0);
glVertex3f(1.0, -1.0, -1.0);
glVertex3f(1.0, -1.0, 1.0);
glVertex3f(-1.0, -1.0, 1.0);
glEnd();
}
void display() // 显示函数
{
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
gluLookAt(cameraPos[0], cameraPos[1], cameraPos[2], cameraPos[0] + cameraFront[0], cameraPos[1] + cameraFront[1], cameraPos[2] + cameraFront[2], cameraUp[0], cameraUp[1], cameraUp[2]);
drawCube();
glutSwapBuffers();
}
void reshape(int width, int height) // 窗口大小变化回调函数
{
glViewport(0, 0, width, height);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
if (width <= height)
{
glOrtho(-2.5, 2.5, -2.5 * (GLfloat)height / (GLfloat)width, 2.5 * (GLfloat)height / (GLfloat)width, -10.0, 10.0);
}
else
{
glOrtho(-2.5 * (GLfloat)width / (GLfloat)height, 2.5 * (GLfloat)width / (GLfloat)height, -2.5, 2.5, -10.0, 10.0);
}
glMatrixMode(GL_MODELVIEW);
}
void keyboard(unsigned char key, int x, int y) // 键盘回调函数
{
float cameraSpeed = 0.1;
switch (key)
{
case 'w': // 前进
cameraPos[0] += cameraSpeed * cameraFront[0];
cameraPos[1] += cameraSpeed * cameraFront[1];
cameraPos[2] += cameraSpeed * cameraFront[2];
break;
case 's': // 后退
cameraPos[0] -= cameraSpeed * cameraFront[0];
cameraPos[1] -= cameraSpeed * cameraFront[1];
cameraPos[2] -= cameraSpeed * cameraFront[2];
break;
case 'a': // 左移
cameraPos[0] -= cameraSpeed * cameraFront[2];
cameraPos[2] += cameraSpeed * cameraFront[0];
break;
case 'd': // 右移
cameraPos[0] += cameraSpeed * cameraFront[2];
cameraPos[2] -= cameraSpeed * cameraFront[0];
break;
}
}
int main(int argc, char **argv) // 主函数
{
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_DOUBLE | GLUT_RGB | GLUT_DEPTH);
glutInitWindowSize(800, 600);
glutCreateWindow("Cube");
glutReshapeFunc(reshape);
glutDisplayFunc(display);
glutKeyboardFunc(keyboard);
glEnable(GL_DEPTH_TEST);
glutMainLoop();
return 0;
}
```
使用方法:
- 编译运行程序,窗口中会显示一个立方体。
- 可以使用 WASD 键控制相机移动,观察立方体不同的视角。
- 使用鼠标拖动窗口大小,可以观察正交投影和透视投影的区别。
相关推荐
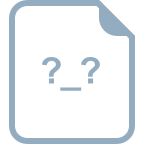














