使用Python的docx库来读取Word文档,通过遍历文档中的段落和run(文本块)来查找红色和加粗文字,然后将结果输出到指定文件中。 对于表格的查找可以使用Python的docx库,通过遍历文档中的表格和单元格来查找表格中的内容,然后将结果输出到指定文件中。 对于超文本链接的查找可以使用Python的docx库,通过遍历文档中的段落和run,查找包含超链接的文本块,然后将超链接和链接地址输出到指定文件中。 统计文档中段落、表格、图片、字符、空格的数量可以使用Python的docx库,通过遍历文档中的元素来统计数量,然后将结果输出到指定文件中。
时间: 2024-02-17 07:01:26 浏览: 24
好的,以下是一些示例代码,可以用于参考:
1. 查找红色和加粗文字:
```python
import docx
doc = docx.Document('example.docx')
output_file = '班级-学号-姓名.txt'
with open(output_file, 'a', encoding='utf-8') as f:
f.write('查找红色和加粗文字\n\n')
for p in doc.paragraphs:
for run in p.runs:
if run.bold and run.font.color.rgb == docx.shared.RGBColor(255, 0, 0):
f.write(f'{run.text}\n')
```
2. 查找表格中的内容:
```python
import docx
doc = docx.Document('example.docx')
output_file = '班级-学号-姓名.txt'
with open(output_file, 'a', encoding='utf-8') as f:
f.write('查找表格中的内容\n\n')
for table in doc.tables:
for row in table.rows:
for cell in row.cells:
for p in cell.paragraphs:
f.write(f'{p.text}\n')
```
3. 查找超文本链接和链接地址:
```python
import docx
doc = docx.Document('example.docx')
output_file = '班级-学号-姓名.txt'
with open(output_file, 'a', encoding='utf-8') as f:
f.write('查找超文本链接和链接地址\n\n')
for p in doc.paragraphs:
for run in p.runs:
if run.element.xml.startswith('<w:hyperlink'):
hyperlink = run.element.xml.split('"')[1]
f.write(f'{run.text}\n{hyperlink}\n')
```
4. 统计文档中段落、表格、图片、字符、空格的数量:
```python
import docx
doc = docx.Document('example.docx')
output_file = '班级-学号-姓名.txt'
num_paragraphs = len(doc.paragraphs)
num_tables = len(doc.tables)
num_images = len(doc.inline_shapes)
num_chars = 0
num_spaces = 0
for p in doc.paragraphs:
num_chars += len(p.text)
num_spaces += p.text.count(' ')
with open(output_file, 'a', encoding='utf-8') as f:
f.write('统计文档中段落、表格、图片、字符、空格的数量\n\n')
f.write(f'段落数量:{num_paragraphs}\n')
f.write(f'表格数量:{num_tables}\n')
f.write(f'图片数量:{num_images}\n')
f.write(f'字符数量:{num_chars}\n')
f.write(f'空格数量:{num_spaces}\n')
```
以上代码仅供参考,具体实现方式可能需要根据具体需求进行修改。
相关推荐
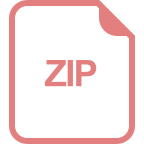
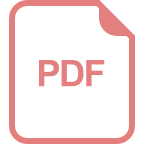













