2、先建立一个职工类Employee,包含数据成员name(姓名),ID(编号),以他为基类,派生出经理类Manager和技术人员类Technician,在经理类中增加数据成员salary(月工资),在技术人员类中增加数据成员wage(代表每小时的工资)和hours(工作时长)。在定义类时,所有类中必须包含构造函数、析构函数、修改和获取所有数据成员的函数,以及虚函数计算职工的工资、输出职工的信息。c++
时间: 2024-02-28 09:55:38 浏览: 86
好的,我来定义一个职工类Employee,并派生出经理类Manager和技术人员类Technician。
```c++
#include <iostream>
#include <string>
using namespace std;
class Employee {
protected:
string name; // 姓名
int ID; // 编号
public:
Employee(string n = "", int id = 0) : name(n), ID(id) {}
virtual ~Employee() {}
virtual double calculatePay() const = 0; // 计算职工的工资
virtual void display() const = 0; // 输出职工的信息
void setName(string n) {
name = n;
}
string getName() const {
return name;
}
void setID(int id) {
ID = id;
}
int getID() const {
return ID;
}
};
class Manager : public Employee {
private:
double salary; // 月工资
public:
Manager(string n = "", int id = 0, double s = 0.0) : Employee(n, id), salary(s) {}
double calculatePay() const {
return salary;
}
void display() const {
cout << "姓名:" << name << endl;
cout << "编号:" << ID << endl;
cout << "月工资:" << salary << endl;
}
void setSalary(double s) {
salary = s;
}
double getSalary() const {
return salary;
}
};
class Technician : public Employee {
private:
double wage; // 每小时的工资
double hours; // 工作时长
public:
Technician(string n = "", int id = 0, double w = 0.0, double h = 0.0) : Employee(n, id), wage(w), hours(h) {}
double calculatePay() const {
return wage * hours;
}
void display() const {
cout << "姓名:" << name << endl;
cout << "编号:" << ID << endl;
cout << "每小时的工资:" << wage << endl;
cout << "工作时长:" << hours << "小时" << endl;
}
void setWage(double w) {
wage = w;
}
double getWage() const {
return wage;
}
void setHours(double h) {
hours = h;
}
double getHours() const {
return hours;
}
};
int main() {
Manager m("张三", 1001, 10000.0);
Technician t("李四", 1002, 100.0, 80.0);
cout << "经理的信息:" << endl;
m.display();
cout << "工资:" << m.calculatePay() << "元" << endl << endl;
cout << "技术人员的信息:" << endl;
t.display();
cout << "工资:" << t.calculatePay() << "元" << endl;
return 0;
}
```
上面的代码中,我们定义了一个职工类Employee,并在其基础上派生出经理类Manager和技术人员类Technician。在Employee类中,我们定义了姓名和编号两个数据成员,以及一个虚函数calculatePay()用于计算职工的工资,一个虚函数display()用于输出职工的信息。在Manager类中,我们增加了月工资这个数据成员,并重写了calculatePay()和display()函数。在Technician类中,我们增加了每小时的工资和工作时长两个数据成员,并同样重写了calculatePay()和display()函数。
在main()函数中,我们分别定义了一个经理对象m和一个技术人员对象t,并输出它们的信息和工资。
运行结果如下:
```
经理的信息:
姓名:张三
编号:1001
月工资:10000
工资:10000元
技术人员的信息:
姓名:李四
编号:1002
每小时的工资:100
工作时长:80小时
工资:8000元
```
希望这个例子可以帮助你理解如何定义一个基类,并在其基础上派生出子类。
阅读全文
相关推荐
















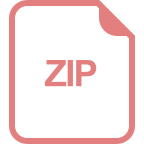
