import java.io.*; public class FileCopy { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.print("请输入源文件名:"); String srcFileName = scanner.nextLine(); System.out.print("请输入目标文件名:"); String destFileName = scanner.nextLine(); try (BufferedInputStream in = new BufferedInputStream(new FileInputStream(srcFileName)); BufferedOutputStream out = new BufferedOutputStream(new FileOutputStream(destFileName))) { byte[] buffer = new byte[1024]; int len; while ((len = in.read(buffer)) != -1) { out.write(buffer, 0, len); } System.out.println("拷贝文件成功!"); } catch (IOException e) { System.out.println("拷贝文件失败:" + e.getMessage()); } 请分析这段代码
时间: 2023-07-15 22:13:57 浏览: 60
这段代码实现了文件拷贝的功能。具体分析如下:
1. 程序从控制台输入源文件名和目标文件名。
2. 使用 try-with-resources 语句来打开源文件和目标文件的输入输出流,以确保在使用完后关闭它们。
3. 定义一个 1024 字节大小的缓冲区 byte[] buffer,用于存储从源文件读取的数据。
4. 使用 while 循环读取源文件中的数据,将其写入目标文件中。如果读取的字节数为 -1,则表示已经读取完整个文件,结束循环。
5. 在读取和写入数据的过程中,如果发生异常,会被 catch 语句捕获。程序将输出错误信息,指示拷贝文件失败。
6. 如果没有发生异常,则表示文件拷贝成功。程序将输出一条成功信息。
总之,这段代码通过缓冲输入输出流和缓冲区的方式,实现了高效的文件拷贝。
相关问题
4.利用文件输入/输出流和缓冲流编写一个实现文件拷贝的程序,源文件名和目标文件名通过Scanner类从控制台获取。
下面是一个实现文件拷贝的程序,可以将一个文件中的内容复制到另一个文件中:
```java
import java.io.*;
public class FileCopy {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入源文件名:");
String sourceFileName = scanner.nextLine();
System.out.print("请输入目标文件名:");
String targetFileName = scanner.nextLine();
try (
FileInputStream fis = new FileInputStream(sourceFileName);
BufferedInputStream bis = new BufferedInputStream(fis);
FileOutputStream fos = new FileOutputStream(targetFileName);
BufferedOutputStream bos = new BufferedOutputStream(fos);
) {
byte[] buffer = new byte[1024];
int len;
while ((len = bis.read(buffer)) != -1) {
bos.write(buffer, 0, len);
}
bos.flush();
System.out.println("文件复制成功!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
上面的代码中,我们使用了输入流和输出流来读取和写入文件,并使用了缓冲流来提高读写效率。在程序运行时,通过Scanner类从控制台获取源文件名和目标文件名,并将源文件中的内容复制到目标文件中。
利用文件输入输出流和缓冲流写一个实现文件拷贝的程序,源文件和目标文件名通过Scanner类从控制台获取
好的,以下是实现文件拷贝的程序:
```
import java.io.*;
public class FileCopy {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入源文件名:");
String sourceFileName = scanner.nextLine();
System.out.print("请输入目标文件名:");
String targetFileName = scanner.nextLine();
try (BufferedInputStream bis = new BufferedInputStream(new FileInputStream(sourceFileName));
BufferedOutputStream bos = new BufferedOutputStream(new FileOutputStream(targetFileName))) {
byte[] buffer = new byte[1024];
int len;
while ((len = bis.read(buffer)) != -1) {
bos.write(buffer, 0, len);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
程序中使用了`BufferedInputStream`和`BufferedOutputStream`来提高文件读写的效率,同时使用了try-with-resources语句来自动关闭流。在读写文件时,每次读取1024字节的数据,然后写入目标文件中。
相关推荐





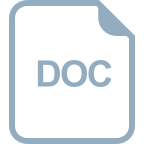
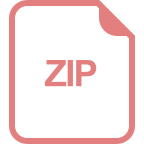
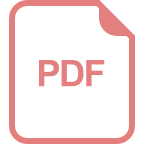