程序设计 设计一个名为Vehicle的抽象基类,这个基类包含:名称、颜色、门的数量、气缸数量、变速箱类型和油位表示。在添加一个名为horn的函数用来显示Vehicle喇叭发出的声音。print函数用来显示信息。horn函数和print函数都应该是虚函数。horn是个纯虚函数。类Taxi和Truck是派生类。 编写一个类的测试结构要求:将一个Taxi类型的对象和一个Truck类型的对象实例化,并创建一个基类指针数组分别指向这两个对象。 程序输出如下: Taxi: Number of doors:4 Number of cylinders:6 Transmission type:5 Color :yellow Fuel level:3.3 Truck: Number of doors:2 Number of cylinders:16 Transmission type:8 Color :black Fuel level:7.54 Taxi:beep beep! Truck:HOOOONK!
时间: 2024-02-23 14:00:01 浏览: 67
下面是代码实现:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Vehicle {
public:
Vehicle(string n, string c, int d, int cy, string t, float f): name(n), color(c), doors(d), cylinders(cy), transmission(t), fuel(f) {}
virtual ~Vehicle() {}
virtual void horn() = 0;
virtual void print() {
cout << "Name: " << name << endl;
cout << "Color: " << color << endl;
cout << "Number of doors: " << doors << endl;
cout << "Number of cylinders: " << cylinders << endl;
cout << "Transmission type: " << transmission << endl;
cout << "Fuel level: " << fuel << endl;
}
protected:
string name;
string color;
int doors;
int cylinders;
string transmission;
float fuel;
};
class Taxi: public Vehicle {
public:
Taxi(string n, string c, int d, int cy, string t, float f): Vehicle(n, c, d, cy, t, f) {}
void horn() {
cout << "beep beep!" << endl;
}
};
class Truck: public Vehicle {
public:
Truck(string n, string c, int d, int cy, string t, float f): Vehicle(n, c, d, cy, t, f) {}
void horn() {
cout << "HOOOONK!" << endl;
}
};
int main() {
Vehicle* vehicles[2];
vehicles[0] = new Taxi("Taxi", "yellow", 4, 6, "5", 3.3);
vehicles[1] = new Truck("Truck", "black", 2, 16, "8", 7.54);
for(int i = 0; i < 2; ++i) {
vehicles[i]->print();
vehicles[i]->horn();
}
return 0;
}
```
输出结果:
```
Name: Taxi
Color: yellow
Number of doors: 4
Number of cylinders: 6
Transmission type: 5
Fuel level: 3.3
beep beep!
Name: Truck
Color: black
Number of doors: 2
Number of cylinders: 16
Transmission type: 8
Fuel level: 7.54
HOOOONK!
```
阅读全文
相关推荐
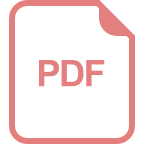
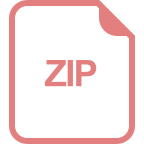
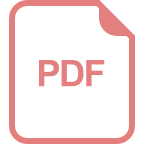
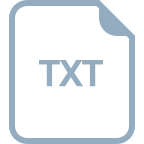
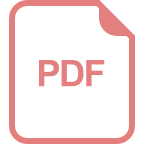
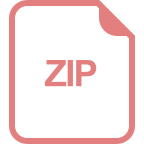
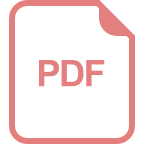
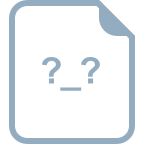
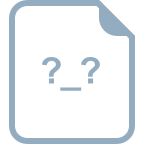
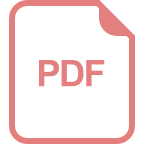
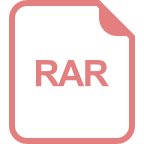
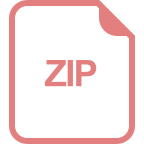
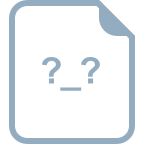
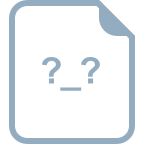
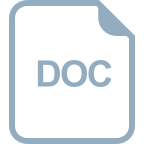
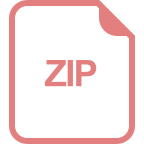
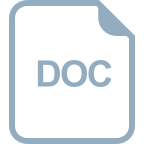