Lvgl+NTPClient做一个动态时钟代码
时间: 2024-01-16 16:02:44 浏览: 204
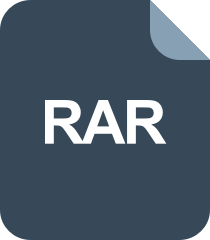
ntp_ntp对时代码_ntpclient_ntp_
以下是使用Lvgl和ESP32的NTPClient库制作动态时钟的示例代码:
```
#include <lvgl.h>
#include <WiFi.h>
#include <NTPClient.h>
#include <WiFiUdp.h>
// Set the WiFi credentials
const char* ssid = "your_SSID";
const char* password = "your_PASSWORD";
// Create a WiFiClient instance to connect to the internet
WiFiClient wifiClient;
// Create a UDP instance to send and receive NTP packets
WiFiUDP ntpUDP;
// Create an NTPClient instance to get the time from NTP servers
NTPClient timeClient(ntpUDP, "pool.ntp.org");
// Create a label to display the time
lv_obj_t* label;
void setup() {
// Initialize LVGL
lv_init();
// Initialize the display
lv_disp_drv_t disp_drv;
lv_disp_drv_init(&disp_drv);
disp_drv.disp_flush = my_disp_flush;
lv_disp_drv_register(&disp_drv);
// Initialize the touch screen
lv_indev_drv_t indev_drv;
lv_indev_drv_init(&indev_drv);
indev_drv.type = LV_INDEV_TYPE_POINTER;
indev_drv.read_cb = my_touchpad_read;
lv_indev_drv_register(&indev_drv);
// Connect to the WiFi network
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
}
// Start the NTP client
timeClient.begin();
// Create the label to display the time
label = lv_label_create(lv_scr_act(), NULL);
lv_label_set_text(label, "00:00:00");
lv_obj_align(label, NULL, LV_ALIGN_CENTER, 0, 0);
// Set the update callback for the time client
timeClient.onNTPSyncEvent([](NTPSyncEvent_t event) {
if (event == timeSyncd) {
// Update the label with the current time
char timeStr[9];
sprintf(timeStr, "%02d:%02d:%02d", timeClient.getHours(), timeClient.getMinutes(), timeClient.getSeconds());
lv_label_set_text(label, timeStr);
lv_obj_align(label, NULL, LV_ALIGN_CENTER, 0, 0);
}
});
}
void loop() {
// Update the time client
timeClient.update();
}
// Implement the display flush function
void my_disp_flush(lv_disp_drv_t* disp_drv, const lv_area_t* area, lv_color_t* color_p) {
// Write the framebuffer to the display
}
// Implement the touchpad read function
bool my_touchpad_read(lv_indev_drv_t* indev_drv, lv_indev_data_t* data) {
// Read the touchpad and update the data
}
```
在此示例代码中,我们首先初始化了LVGL,并为显示器和触摸屏创建了驱动程序。然后,我们连接到WiFi网络,并创建了一个NTPClient实例来获取当前时间。接下来,我们创建了一个标签来显示时间,并将其对齐到屏幕中心。最后,我们设置了一个回调函数,用于更新标签以显示当前时间。在主循环中,我们更新时间客户端,以便它可以更新当前时间。
阅读全文
相关推荐
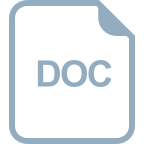
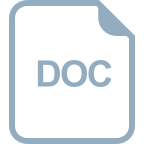
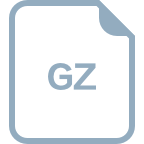
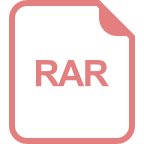
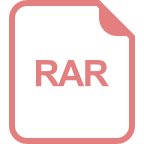
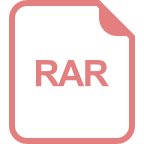
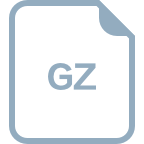
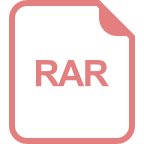
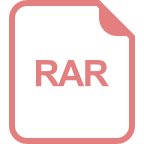
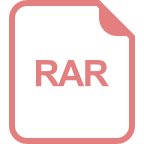
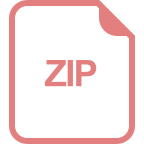






