javafx登录注册页面
时间: 2023-07-30 09:07:21 浏览: 167
以下是一个简单的JavaFX登录注册页面的示例代码:
```java
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.control.PasswordField;
import javafx.scene.control.TextField;
import javafx.scene.layout.GridPane;
import javafx.scene.paint.Color;
import javafx.scene.text.Font;
import javafx.scene.text.FontWeight;
import javafx.scene.text.Text;
import javafx.stage.Stage;
public class LoginRegistrationPage extends Application {
@Override
public void start(Stage primaryStage) throws Exception {
primaryStage.setTitle("Login / Registration Page");
// Create a GridPane layout
GridPane grid = new GridPane();
grid.setAlignment(Pos.CENTER);
grid.setHgap(10);
grid.setVgap(10);
grid.setPadding(new Insets(25, 25, 25, 25));
// Add a Text node
Text scenetitle = new Text("Welcome");
scenetitle.setFont(Font.font("Tahoma", FontWeight.NORMAL, 20));
grid.add(scenetitle, 0, 0, 2, 1);
// Add a Label and TextField for username
Label userName = new Label("User Name:");
grid.add(userName, 0, 1);
TextField userTextField = new TextField();
grid.add(userTextField, 1, 1);
// Add a Label and PasswordField for password
Label pw = new Label("Password:");
grid.add(pw, 0, 2);
PasswordField pwBox = new PasswordField();
grid.add(pwBox, 1, 2);
// Add a login Button
Button btn = new Button("Login");
HBox hbBtn = new HBox(10);
hbBtn.setAlignment(Pos.BOTTOM_RIGHT);
hbBtn.getChildren().add(btn);
grid.add(hbBtn, 1, 4);
// Add a registration Button
Button registrationBtn = new Button("Register");
HBox registrationHbBtn = new HBox(10);
registrationHbBtn.setAlignment(Pos.BOTTOM_RIGHT);
registrationHbBtn.getChildren().add(registrationBtn);
grid.add(registrationHbBtn, 1, 5);
// Add a message Text node
final Text actiontarget = new Text();
grid.add(actiontarget, 1, 6);
// Handle login Button action
btn.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent e) {
if (userTextField.getText().equals("username") && pwBox.getText().equals("password")) {
actiontarget.setFill(Color.GREEN);
actiontarget.setText("Login successful!");
} else {
actiontarget.setFill(Color.RED);
actiontarget.setText("Invalid username or password.");
}
}
});
// Handle registration Button action
registrationBtn.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent e) {
// TODO: Implement registration functionality
actiontarget.setFill(Color.BLUE);
actiontarget.setText("Registration page coming soon!");
}
});
// Show the scene
Scene scene = new Scene(grid, 300, 275);
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
```
这个示例代码创建了一个简单的JavaFX登录注册页面,包括一个欢迎文本、用户名和密码输入框、登录和注册按钮以及一个消息文本。在点击登录按钮时,它会检查输入的用户名和密码是否正确,并在消息文本中显示相应的结果。在点击注册按钮时,它会显示一个提示消息,表示注册页面即将推出。你可以根据自己的需求自定义该页面的样式和功能。
阅读全文
相关推荐

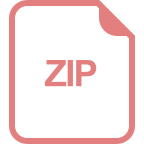
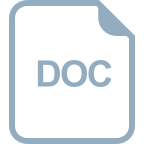




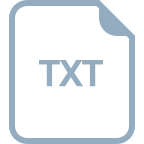
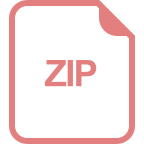
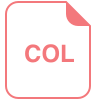
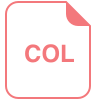
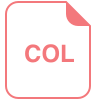
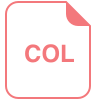


