能不能基于vue3 给出通过按钮放大或缩小D3.js 视图内 svg 的代码
时间: 2024-02-28 20:57:42 浏览: 247
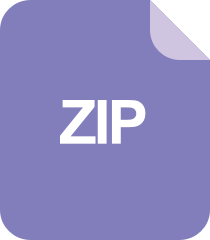
Vue2 D3.js demo

当使用 Vue 3 结合 D3.js 来实现可视化时,经常需要通过按钮来放大或缩小 SVG 视图。下面是一个基于 Vue 3 和 D3.js 的通过按钮放大或缩小 SVG 视图的示例代码:
```
<template>
<div>
<div ref="container">
<svg :width="width" :height="height" ref="svg">
<g :transform="`translate(${margin.left}, ${margin.top})`" ref="g">
<circle cx="50" cy="50" r="50" fill="red"/>
</g>
</svg>
</div>
<div>
<button @click="zoomIn">放大</button>
<button @click="zoomOut">缩小</button>
</div>
</div>
</template>
<script>
import * as d3 from 'd3';
import { ref, onMounted } from 'vue';
export default {
setup() {
const container = ref(null);
const svg = ref(null);
const g = ref(null);
const width = 500;
const height = 500;
const margin = { top: 20, right: 20, bottom: 20, left: 20 };
const zoom = d3.zoom().on('zoom', zoomed);
function zoomed() {
const transform = d3.event.transform;
g.value.setAttribute('transform', transform);
}
function zoomIn() {
const scale = d3.zoomTransform(svg.value).scale();
const newScale = scale + 0.1;
zoomTo(newScale);
}
function zoomOut() {
const scale = d3.zoomTransform(svg.value).scale();
const newScale = Math.max(0.1, scale - 0.1);
zoomTo(newScale);
}
function zoomTo(scale) {
const newTransform = d3.zoomIdentity.scale(scale);
d3.select(svg.value)
.transition()
.duration(500)
.call(zoom.transform, newTransform);
}
onMounted(() => {
d3.select(container.value)
.call(zoom)
.call(zoom.transform, d3.zoomIdentity.translate(margin.left, margin.top));
});
return {
container,
svg,
g,
width,
height,
margin,
zoomIn,
zoomOut,
};
},
};
</script>
```
在这个示例代码中,我们使用了 Vue 3 的 `ref` 函数来声明了一个指向容器元素、SVG 元素和 `g` 元素的引用 `container`、`svg` 和 `g`,并且定义了 SVG 视图的大小 `width` 和 `height`,以及 SVG 视图内部的边距 `margin`。
在 `setup` 函数中,我们使用 D3.js 的 `zoom` 函数来创建一个缩放操作,并将其绑定到容器元素上。在 `zoomed` 函数中,我们使用当前缩放的变换矩阵来更新 `g` 元素的变换属性。
同时,我们定义了 `zoomIn` 和 `zoomOut` 函数来放大和缩小 SVG 视图,分别计算当前缩放比例并进行相应的缩放操作。在 `zoomTo` 函数中,我们使用 `transition` 函数来实现缓动效果,并使用 `call` 函数将缩放操作应用到 SVG 元素上。
最后,在模板中,我们添加了两个按钮来触发放大和缩小操作,并使用 `@click` 事件绑定相应的函数。
注意:在使用 Vue 3 和 D3.js 结合实现可视化的过程中,需要注意缩放操作的兼容性和性能问题。
阅读全文
相关推荐
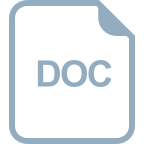
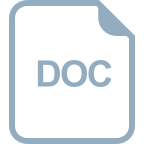

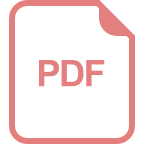
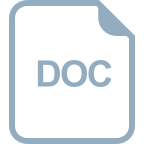
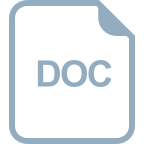
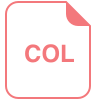
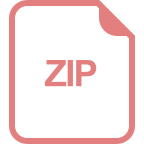
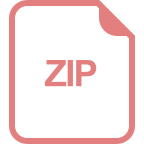
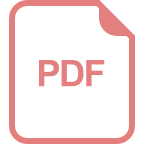
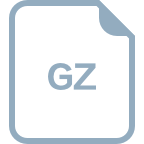
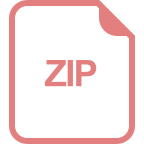
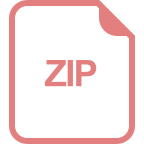
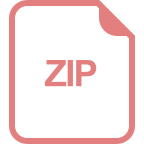
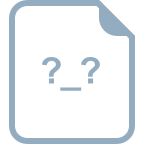
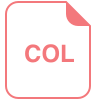

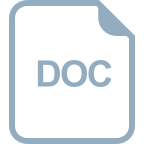
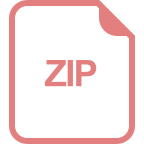