用python pytochj写一个 5个数据320x 240x 1维度输入 包含 卷积+convLSTM 卷积操作块+conv+BatchNorm2d+sigmoid+分割输出维度 320x240x1
时间: 2023-12-30 17:06:26 浏览: 94
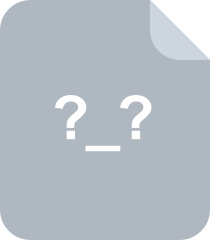
卷积python代码

好的,我明白了。关于这个问题,我可以告诉你,可以用PyTorch的nn模块来实现卷积神经网络和LSTM的结合。
首先定义一个包含卷积和LSTM的网络层,代码如下:
```
import torch
import torch.nn as nn
class ConvLSTMLayer(nn.Module):
def __init__(self, input_size, hidden_size, kernel_size):
super(ConvLSTMLayer, self).__init__()
self.hidden_size = hidden_size
self conv = nn.Conv2d(input_size + hidden_size, hidden_size, kernel_size, padding=kernel_size // 2)
self.lstm = nn.LSTMCell(hidden_size, hidden_size)
def forward(self, input, hidden):
hx, cx = hidden
combined = torch.cat((input, hx), dim=1)
combined_conv = self.conv(combined)
hx, cx = self.lstm(combined_conv, (hx, cx))
return hx, cx
```
然后定义一个卷积操作块,代码如下:
```
class ConvBlock(nn.Module):
def __init__(self, in_channels, out_channels):
super(ConvBlock, self).__init__()
self.conv1 = nn.Conv2d(in_channels, out_channels, kernel_size=3, stride=1, padding=1, bias=False)
self.bn1 = nn.BatchNorm2d(out_channels)
self.relu = nn.ReLU(inplace=True)
def forward(self, x):
x = self.conv1(x)
x = self.bn1(x)
x = self.relu(x)
return x
```
接下来是定义一个包含sigmoid的输出层,代码如下:
```
class OutputLayer(nn.Module):
def __init__(self, in_channels):
super(OutputLayer, self).__init__()
self.conv = nn.Conv2d(in_channels, 1, kernel_size=1, stride=1, bias=False)
self.sigmoid = nn.Sigmoid()
def forward(self, x):
x = self.conv(x)
x = self.sigmoid(x)
return x
```
最后,定义整个模型,代码如下:
```
class Model(nn.Module):
def __init__(self):
super(Model, self).__init__()
self.conv1 = ConvBlock(1, 64)
self.conv2 = ConvBlock(64, 128)
self.conv3 = ConvBlock(128, 256)
self.conv_lstm1 = ConvLSTMLayer(256, 256, 3)
self.conv_lstm2 = ConvLSTMLayer(256, 256, 3)
self.output_layer = OutputLayer(256)
def forward(self, x):
batch_size, timesteps, C, H, W = x.size()
device = x.device
hidden_state = torch.zeros(batch_size, 256, H // 8, W // 8).to(device)
cell_state = torch.zeros(batch_size, 256, H // 8, W // 8).to(device)
for i in range(timesteps):
x_ = x[:, i, :, :, :]
x_ = self.conv1(x_)
x_ = self.conv2(x_)
x_ = self.conv3(x_)
hidden_state, cell_state = self.conv_lstm1(x_, (hidden_state, cell_state))
hidden_state, cell_state = self.conv_lstm2(hidden_state, (hidden_state, cell_state))
out = self.output_layer(hidden_state)
return out
```
这个模型包含了五个输入数据,每个数据都是320x240x1的,输入到模型中,经过卷积神经网络和LSTM的结合,再经过输出层的处理,最终输出320x240x1的分割结果。
阅读全文
相关推荐
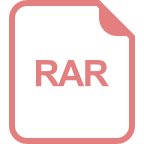
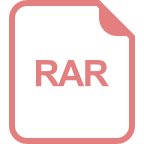
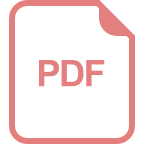
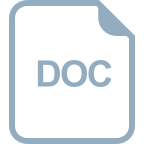
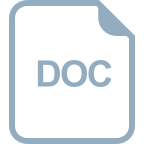
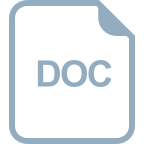
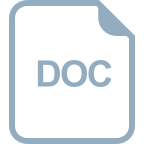

