给出DFS运用stack实现的C++代码
时间: 2023-06-20 16:03:30 浏览: 107
以下是使用栈实现 DFS 的 C++ 代码:
```c++
#include <iostream>
#include <stack>
#include <vector>
using namespace std;
void dfs(int start, vector<int> graph[], bool visited[]) {
stack<int> s;
s.push(start);
visited[start] = true;
while (!s.empty()) {
int current = s.top();
s.pop();
cout << current << " ";
for (int i = 0; i < graph[current].size(); i++) {
int next = graph[current][i];
if (!visited[next]) {
visited[next] = true;
s.push(next);
}
}
}
}
int main() {
int n = 5; // number of nodes
vector<int> graph[n];
// add edges to the graph
graph[0].push_back(1);
graph[0].push_back(2);
graph[1].push_back(2);
graph[2].push_back(0);
graph[2].push_back(3);
graph[3].push_back(3);
bool visited[n];
for (int i = 0; i < n; i++) {
visited[i] = false;
}
cout << "DFS traversal starting from node 2: ";
dfs(2, graph, visited);
return 0;
}
```
该代码使用了一个栈来实现深度优先搜索。首先将起点加入栈中并标记为已访问,然后不断地从栈中取出顶部元素,访问它并将其未访问的邻居加入栈中。重复这个过程直到栈为空。在这个例子中,我们从节点 2 开始进行深度优先搜索。
阅读全文
相关推荐



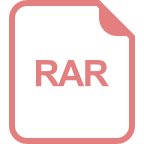
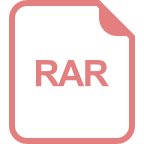













