ArcGIS engineeC#慢速演示点从一个坐标移动向另一个坐标的轨迹过程
时间: 2023-09-16 22:12:53 浏览: 71
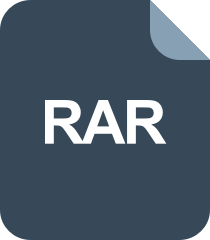
arcengine中实现点的移动
首先,我们需要创建一个ArcGIS Engine的C#项目,并添加一个MapControl控件。
接下来,我们需要定义两个点,即起点和终点。可以使用IPoint接口来表示这两个点。例如:
```csharp
IPoint startPoint = new PointClass();
startPoint.X = 100;
startPoint.Y = 100;
IPoint endPoint = new PointClass();
endPoint.X = 200;
endPoint.Y = 200;
```
然后,我们可以使用IMarkerSymbol接口来定义一个标记符号,表示移动的点。例如:
```csharp
IMarkerSymbol markerSymbol = new SimpleMarkerSymbolClass();
markerSymbol.Size = 10;
markerSymbol.Style = esriSimpleMarkerStyle.esriSMSCircle;
```
接下来,我们可以使用IElement接口来创建一个元素,并将标记符号与起点关联。例如:
```csharp
IElement element = new MarkerElementClass();
element.Geometry = startPoint;
element.Symbol = markerSymbol;
```
然后,我们可以将元素添加到地图中。例如:
```csharp
IMap map = axMapControl1.Map;
IElementContainer elementContainer = map as IElementContainer;
elementContainer.AddElement(element, 0);
axMapControl1.Refresh();
```
接下来,我们可以使用IPointCollection接口来获取起点和终点之间的所有点,并依次移动元素。例如:
```csharp
IPointCollection pointCollection = new PathClass();
pointCollection.AddPoint(startPoint);
pointCollection.AddPoint(endPoint);
double distance = startPoint.Distance(endPoint);
double step = distance / 100;
double currentDistance = 0;
while (currentDistance < distance)
{
for (int i = 1; i < pointCollection.PointCount; i++)
{
IPoint fromPoint = pointCollection.get_Point(i - 1);
IPoint toPoint = pointCollection.get_Point(i);
double segmentDistance = fromPoint.Distance(toPoint);
double segmentStep = step;
while (segmentStep < segmentDistance)
{
IPoint currentPoint = new PointClass();
currentPoint.X = fromPoint.X + (toPoint.X - fromPoint.X) * segmentStep / segmentDistance;
currentPoint.Y = fromPoint.Y + (toPoint.Y - fromPoint.Y) * segmentStep / segmentDistance;
element.Geometry = currentPoint;
axMapControl1.Refresh();
segmentStep += step;
currentDistance += step;
System.Threading.Thread.Sleep(50);
}
}
}
```
上面的代码中,我们首先计算起点和终点之间的距离,然后将其分为100个步骤。然后,我们依次遍历起点和终点之间的每个线段,并在每个线段上移动元素。我们通过计算每个线段的长度和步骤来确定当前点的位置。在每次移动后,我们使用System.Threading.Thread.Sleep方法来暂停50毫秒,以便使移动看起来更平滑。
最后,我们可以在移动结束后将元素从地图中删除。例如:
```csharp
elementContainer.DeleteElement(element);
axMapControl1.Refresh();
```
阅读全文
相关推荐
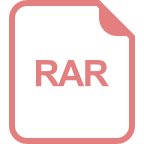
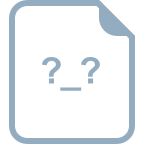
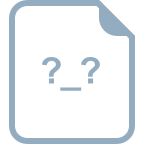
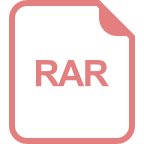
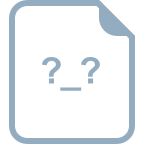
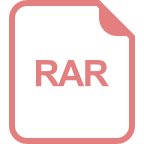
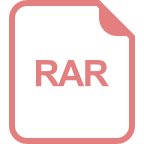
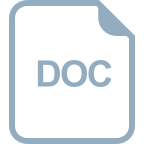
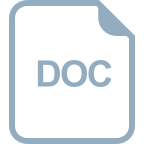
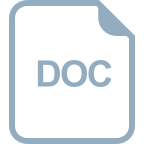
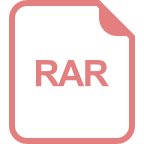
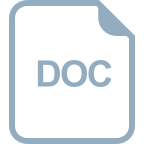
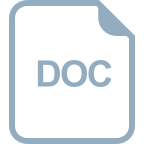
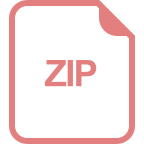
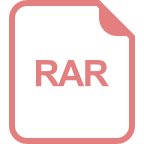
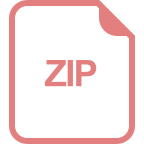
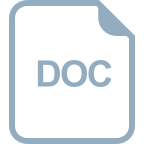