Python中求字符串中最长的单词
时间: 2023-04-08 19:05:25 浏览: 349
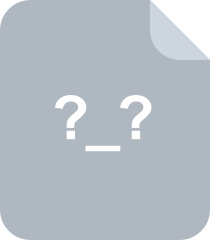
查找字符串最长的单词

可以使用split()函数将字符串分割成单词列表,然后遍历列表找到最长的单词即可。以下是示例代码:
```python
def find_longest_word(s):
words = s.split()
longest_word = ""
for word in words:
if len(word) > len(longest_word):
longest_word = word
return longest_word
s = "Python is a powerful and easy-to-learn programming language."
longest_word = find_longest_word(s)
print("The longest word is:", longest_word)
```
输出结果为:
```
The longest word is: programming
```
阅读全文
相关推荐
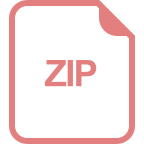


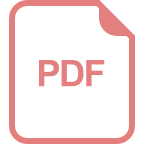












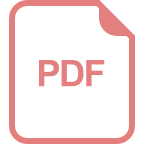