编写Linux应用程序,利用POSIX API在Linux系统上编写应用程序,实现cp命令的部分功能,主要实现文件到文件的复制功能(执行“cp 源文件路径 目标文件路径”实现的功能)。
时间: 2024-02-24 21:59:11 浏览: 94
下面是一个使用POSIX API在Linux系统上编写的cp命令部分功能的示例代码。它可以实现文件到文件的复制功能,类似于执行"cp 源文件路径 目标文件路径"这样的命令。请注意,这只是一个简单的示例代码,没有进行错误处理和边界情况的检查。
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
#define BUFFER_SIZE 1024
int main(int argc, char *argv[]) {
int source_file, target_file;
ssize_t read_bytes, write_bytes;
char buffer[BUFFER_SIZE];
// 检查参数数量
if (argc != 3) {
printf("Usage: %s source_file target_file\n", argv[0]);
exit(EXIT_FAILURE);
}
// 打开源文件
source_file = open(argv[1], O_RDONLY);
if (source_file == -1) {
printf("Error opening source file %s\n", argv[1]);
exit(EXIT_FAILURE);
}
// 创建目标文件
target_file = open(argv[2], O_WRONLY | O_CREAT | O_TRUNC, 0666);
if (target_file == -1) {
printf("Error creating target file %s\n", argv[2]);
exit(EXIT_FAILURE);
}
// 读取源文件并写入目标文件
while ((read_bytes = read(source_file, buffer, BUFFER_SIZE)) > 0) {
write_bytes = write(target_file, buffer, read_bytes);
if (write_bytes != read_bytes) {
printf("Error writing to target file %s\n", argv[2]);
exit(EXIT_FAILURE);
}
}
// 关闭文件
close(source_file);
close(target_file);
return 0;
}
```
在这个示例代码中,我们使用了POSIX API中的open、read、write和close函数来打开、读取、写入和关闭文件。我们还使用了fcntl.h头文件中的一些常量来指定文件的打开方式和权限。最后,在main函数中,我们检查了参数数量,打开了源文件和目标文件,以及将源文件的内容复制到目标文件中。
阅读全文
相关推荐










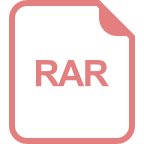







