react中的setState什么情况下同步什么情况下异步
时间: 2024-05-05 11:21:30 浏览: 172
在 React 中,setState 可以是同步的,也可以是异步的,这取决于它的使用场景。
情况一:在 React 的生命周期函数(componentDidMount、componentDidUpdate、componentWillUnmount)以及 React 事件处理函数中,setState 是同步的。
例如,在以下代码中,setState 是同步的:
```
class Example extends React.Component {
constructor(props) {
super(props);
this.state = { count: 0 };
}
componentDidMount() {
this.setState({ count: this.state.count + 1 });
console.log(this.state.count); // 输出 1
}
render() {
return <div>{this.state.count}</div>;
}
}
```
情况二:在 setTimeout、setInterval、Promise 等异步函数中,setState 是异步的。
例如,在以下代码中,setState 是异步的:
```
class Example extends React.Component {
constructor(props) {
super(props);
this.state = { count: 0 };
}
handleClick() {
setTimeout(() => {
this.setState({ count: this.state.count + 1 });
console.log(this.state.count); // 输出 0
}, 1000);
}
render() {
return <button onClick={this.handleClick.bind(this)}>Click</button>;
}
}
```
在异步场景下,React 会将多个 setState 合并为一个更新,以提高性能。因此,如果需要使用最新的 state 值,可以在 setState 中传递一个函数,而不是一个对象。
例如,在以下代码中,setState 中使用函数的方式可以确保获取到最新的 state 值:
```
class Example extends React.Component {
constructor(props) {
super(props);
this.state = { count: 0 };
}
handleClick() {
setTimeout(() => {
this.setState((prevState) => ({ count: prevState.count + 1 }));
console.log(this.state.count); // 输出 1
}, 1000);
}
render() {
return <button onClick={this.handleClick.bind(this)}>Click</button>;
}
}
```
阅读全文
相关推荐
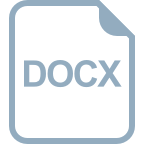
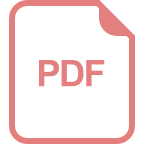

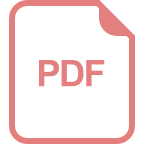
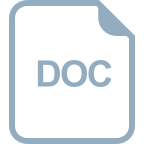
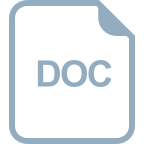
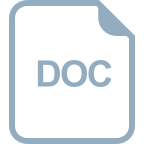
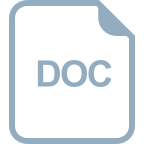
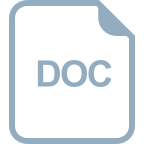






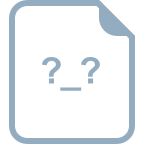
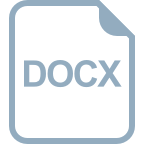