用Java和sql server做一个商品管理系统的程序
时间: 2024-01-21 14:16:20 浏览: 68
好的,以下是一个简单的商品管理系统的Java程序示例。该程序使用Java Swing作为GUI库,使用SQL Server作为后端数据库。
首先,我们需要创建一个数据库表来存储商品信息。以下是一个示例的SQL Server表定义:
```sql
CREATE TABLE products (
id INT PRIMARY KEY IDENTITY,
name VARCHAR(50) NOT NULL,
price DECIMAL(10, 2) NOT NULL,
quantity INT NOT NULL
);
```
然后,我们将使用Java JDBC API来连接SQL Server数据库并执行查询和更新操作。
```java
import java.sql.*;
public class ProductManager {
private static final String DB_URL = "jdbc:sqlserver://localhost:1433;databaseName=mydb;";
private static final String USER = "myuser";
private static final String PASS = "mypassword";
public static void main(String[] args) {
try (Connection conn = DriverManager.getConnection(DB_URL, USER, PASS)) {
System.out.println("Connected to database!");
// create a new product
createProduct(conn, "iPhone 12 Pro", 999.99, 10);
// print all products
printProducts(conn);
// update the price of a product
updateProductPrice(conn, 1, 899.99);
// delete a product
deleteProduct(conn, 2);
} catch (SQLException e) {
System.out.println(e.getMessage());
}
}
private static void createProduct(Connection conn, String name, double price, int quantity) throws SQLException {
String sql = "INSERT INTO products(name, price, quantity) VALUES (?, ?, ?)";
try (PreparedStatement statement = conn.prepareStatement(sql)) {
statement.setString(1, name);
statement.setDouble(2, price);
statement.setInt(3, quantity);
int rowsInserted = statement.executeUpdate();
if (rowsInserted > 0) {
System.out.println("A new product was inserted successfully!");
}
}
}
private static void printProducts(Connection conn) throws SQLException {
String sql = "SELECT * FROM products";
try (Statement statement = conn.createStatement();
ResultSet result = statement.executeQuery(sql)) {
while (result.next()) {
int id = result.getInt("id");
String name = result.getString("name");
double price = result.getDouble("price");
int quantity = result.getInt("quantity");
System.out.println(id + ", " + name + ", " + price + ", " + quantity);
}
}
}
private static void updateProductPrice(Connection conn, int id, double price) throws SQLException {
String sql = "UPDATE products SET price = ? WHERE id = ?";
try (PreparedStatement statement = conn.prepareStatement(sql)) {
statement.setDouble(1, price);
statement.setInt(2, id);
int rowsUpdated = statement.executeUpdate();
if (rowsUpdated > 0) {
System.out.println("The product was updated successfully!");
}
}
}
private static void deleteProduct(Connection conn, int id) throws SQLException {
String sql = "DELETE FROM products WHERE id = ?";
try (PreparedStatement statement = conn.prepareStatement(sql)) {
statement.setInt(1, id);
int rowsDeleted = statement.executeUpdate();
if (rowsDeleted > 0) {
System.out.println("The product was deleted successfully!");
}
}
}
}
```
这个程序使用`createProduct`方法来创建一个新的商品,使用`printProducts`方法来打印所有商品,使用`updateProductPrice`方法来更新商品价格,使用`deleteProduct`方法来删除商品。这些方法都使用Java JDBC API来执行SQL查询和更新操作。
注意,为了运行这个程序,你需要下载并安装SQL Server JDBC驱动程序,并将其添加到你的Java类路径中。
这只是一个简单的示例程序,你可能需要根据你的具体需求进行修改和扩展。希望这可以帮助到你。
阅读全文
相关推荐
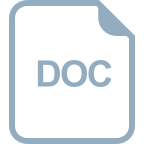
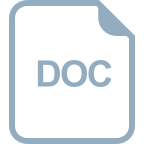
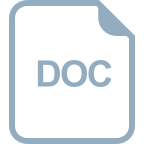
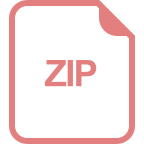
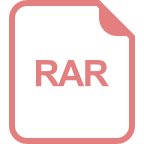
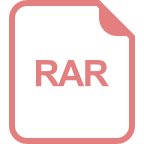

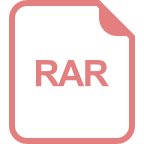
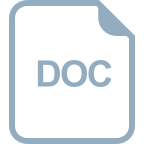
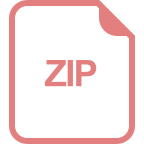
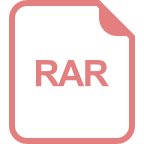


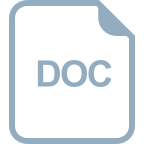
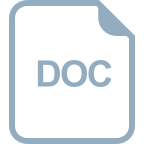