写一个商品管理系统个代码使用Java和sql server
时间: 2024-01-21 18:16:12 浏览: 25
好的,以下是一个简单的商品管理系统的Java代码,使用了sql server数据库:
```java
import java.sql.*;
public class ProductManagementSystem {
static final String DB_URL = "jdbc:sqlserver://localhost:1433;databaseName=ProductDB";
static final String USER = "username";
static final String PASS = "password";
public static void main(String[] args) {
Connection conn = null;
Statement stmt = null;
try {
Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver");
System.out.println("Connecting to database...");
conn = DriverManager.getConnection(DB_URL,USER,PASS);
System.out.println("Creating statement...");
stmt = conn.createStatement();
//创建商品表
String sql = "CREATE TABLE Products " +
"(id INTEGER not NULL, " +
" name VARCHAR(255), " +
" price DOUBLE, " +
" PRIMARY KEY ( id ))";
stmt.executeUpdate(sql);
System.out.println("Product table created successfully.");
//添加商品
sql = "INSERT INTO Products (id, name, price) " +
"VALUES (1, 'Product 1', 10.99)";
stmt.executeUpdate(sql);
sql = "INSERT INTO Products (id, name, price) " +
"VALUES (2, 'Product 2', 20.99)";
stmt.executeUpdate(sql);
sql = "INSERT INTO Products (id, name, price) " +
"VALUES (3, 'Product 3', 30.99)";
stmt.executeUpdate(sql);
System.out.println("Products added successfully.");
//查询所有商品
sql = "SELECT id, name, price FROM Products";
ResultSet rs = stmt.executeQuery(sql);
//打印查询结果
while(rs.next()){
int id = rs.getInt("id");
String name = rs.getString("name");
double price = rs.getDouble("price");
System.out.print("ID: " + id);
System.out.print(", Name: " + name);
System.out.println(", Price: " + price);
}
rs.close();
stmt.close();
conn.close();
} catch (SQLException se) {
se.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (stmt != null) stmt.close();
} catch (SQLException se2) {
}
try {
if (conn != null) conn.close();
} catch (SQLException se) {
se.printStackTrace();
}
}
System.out.println("Goodbye!");
}
}
```
这个系统使用了sql server数据库,并创建了一个名为ProductDB的数据库,其中包含一个名为Products的表格,用于存储商品的信息。在这个系统中,你可以添加、删除和查询商品的信息。
相关推荐
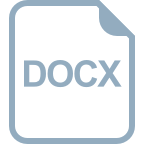














